Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial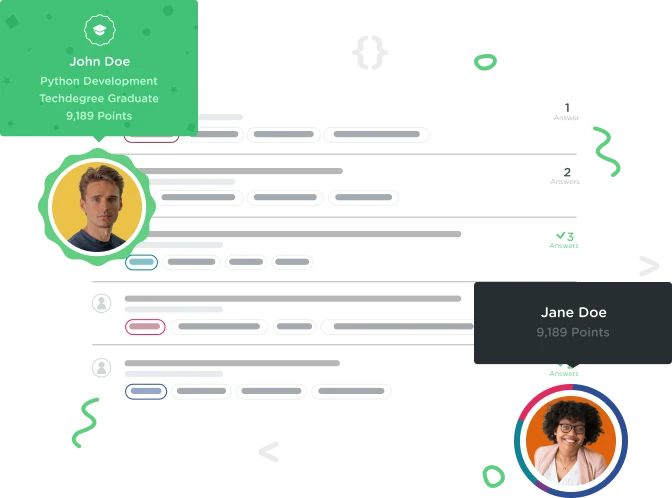

Janis Sauss
4,672 PointsWhy do i get - Exception in thread "main" java.lang.NullPointerException?
When I run the code accordingly to the video I get this error:
Exception in thread "main" java.lang.NullPointerException
at com.teamtreehouse.Treet.compareTo(Treet.java:28)
at java.util.ComparableTimSort.countRunAndMakeAscending(ComparableTimSo
rt.java:320)
at java.util.ComparableTimSort.sort(ComparableTimSort.java:188)
at java.util.Arrays.sort(Arrays.java:1246)
at Example.main(Example.java:26)
Why so?

Janis Sauss
4,672 PointsExample.java
import java.util.Arrays;
import java.util.Date;
import com.teamtreehouse.Treet;
public class Example {
public static void main(String[] args) {
Treet treet = new Treet(
"craigsdennis",
"Want to be famous? Simply tweet about Java and use " +
"the hashtag #treet. I'll use your tweet in a new " +
"@treehouse course about data structures.",
new Date(1421849732000L)
);
Treet secondTreet = new Treet(
"journeytocode",
"@treehouse makes learning Java sooooo fun! #treet",
new Date(1421878767000L)
);
System.out.printf("This is a new Treet: %s %n", treet);
System.out.println("The word are:");
for (String word: treet.getWords()) {
System.out.println(word);
}
Treet[] treets={treet,secondTreet};
Arrays.sort(treets);
for(Treet exampleTreet: treets) {
System.out.println(exampleTreet);
}
}
}

Janis Sauss
4,672 PointsTreet.java:
package com.teamtreehouse;
import java.util.Date;
public class Treet implements Comparable {
private String mAuthor;
private String mDescription;
private Date mCreationDate;
public Treet (String author, String description, Date creationDate) {
mAuthor=author;
mDescription=description;
mCreationDate=mCreationDate;
}
@Override
public String toString() {
return String.format("Treet: \" %s \" by %s on %s", mDescription, mAuthor, mCreationDate);
}
@Override
public int compareTo(Object obj) {
Treet other = (Treet) obj;
if (equals(other)) {
return 0;
}
int dateCmp=mCreationDate.compareTo(other.mCreationDate);
if (dateCmp==0) {
return mDescription.compareTo(other.mDescription);
}
return dateCmp;
}
public String getAuthor () {
return mAuthor;
}
public String getDescription () {
return mAuthor;
}
public Date getCreationDate () {
return mCreationDate;
}
public String[] getWords() {
return mDescription.toLowerCase().split("[^\\w#@']+");
}
}
3 Answers
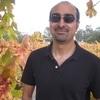
Kourosh Raeen
23,733 PointsThe problem is in the Treet constructor. Change
mCreationDate = mCreationDate;
to
mCreationDate = creationDate;
By the way, the line of code you had for defining an array of type Treet using curly braces was correct. So make sure to change it back to:
Treet[] treets = {treet,secondTreet};

Janis Sauss
4,672 PointsThank you. That did the trick. When somebody else takes a fresh look on the code it is easier to find the mistake.

Sean Hayes
Courses Plus Student 8,106 PointsTry changing:
Treet[] treets={treet,secondTreet};
to...
Treet[] treets=(treet,secondTreet);
When defining an array, you need to use parentheses instead of curly brackets

Janis Sauss
4,672 PointsBut then is shows something like this:
Example.java:31: error: ')' expected
Treet[] treets=(treet, secondTreet);
^
Example.java:31: error: ';' expected
Treet[] treets=(treet, secondTreet);
^
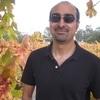
Kourosh Raeen
23,733 PointsThe array declaration & initialization using curly braces is correct. This is a short cut syntax that allows you to both create and initialize the array in one line:
https://docs.oracle.com/javase/tutorial/java/nutsandbolts/arrays.html

Sean Hayes
Courses Plus Student 8,106 PointsTake a look at: http://stackoverflow.com/questions/5364278/creating-an-array-of-objects-in-java
- Declare the Treets array at the top of your main function, with a size of 2: Treet[] treets = new Treet[2]
- Modify the existing declarations for treet and secondTreet to look like: treets[0] = new Treet( blah, blah, blah);
Everything else should run the same. This works when you are only using a fixed number of Treets. If you want to create a list that grows, look into ArrayList. Hope this helps
Sean Hayes
Courses Plus Student 8,106 PointsSean Hayes
Courses Plus Student 8,106 PointsCan you copy and paste the code in here so we can look over it?