Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial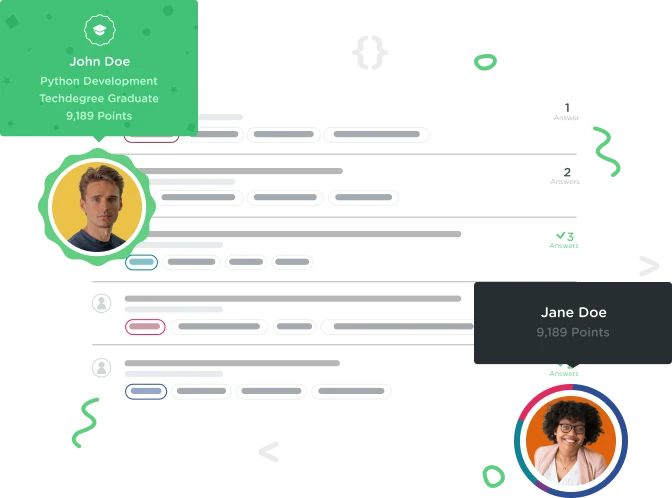

veronikapost
2,552 PointsWhy do I get undefined results when using index in for each() ?
I am trying to take an advantage of the following syntax according to MDN (https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/forEach#Syntax) :
arr.forEach(function callback(currentValue [, index [, array]]) { //your iterator }[, thisArg]);
I am trying to use an index parameter as an index for my new array. My assumption is that forEach will take the first value of 1 and index of 0, and will replace times[0] with the value of 1 * 5, but something is not working as I'm expecting it to..
const numbers = [1,2,3,4,5,6,7,8,9,10];
let times5 = [];
// times5 should be: [5,10,15,20,25,30,35,40,45,50]
// Write your code below
times5 = numbers.forEach(function (number, index) {
times5[index] = number * 5;
});
2 Answers

Vitaly Khe
7,160 PointsHi Veronika,
The following code calls method forEach for an array of numbers.
numbers.forEach(function (number, index) {
times5[index] = number * 5;
});
this code fills up your times5 array correctly. But method forEach returns UNDEFINED after execution. You can see it the documentation by link on MDN.
So, after your array is filled up and cycle inside { } exited, you assign times5 a new value of undefined like by this: times5 = array.forEach(...){...};
look what happens by adding a console.log inside a cycle{} and finally after a cycle:
const numbers = [1,2,3,4,5,6,7,8,9,10];
let times5 = [];
times5 = numbers.forEach(function (number, index) {
times5[index] = number * 5;
console.log(times5);
});
console.log(times5);
[ 5 ]
[ 5, 10 ]
[ 5, 10, 15 ]
[ 5, 10, 15, 20 ]
[ 5, 10, 15, 20, 25 ]
[ 5, 10, 15, 20, 25, 30 ]
[ 5, 10, 15, 20, 25, 30, 35 ]
[ 5, 10, 15, 20, 25, 30, 35, 40 ]
[ 5, 10, 15, 20, 25, 30, 35, 40, 45 ]
[ 5, 10, 15, 20, 25, 30, 35, 40, 45, 50 ]
undefined
Have a good day!

Vitaly Khe
7,160 PointsHi
Pls check the return value for Array.forEach by your link. Other words, you are re-writing times5 by a return value of foreach method. Correct code:
const numbers = [1,2,3,4,5,6,7,8,9,10];
let times5 = [];
numbers.forEach(function (number, index) {
times5[index] = number * 5;
});

veronikapost
2,552 PointsHi Vitaly Khe,
Thank you for your reply. I thought I am supposed to re-write times5 array, since it is declared as an empty array. I just don't understand why I cannot assign the values that I am returning to an array (to put all the new values in times5 array)?
veronikapost
2,552 Pointsveronikapost
2,552 PointsHi Vitaly Khe,
Thank you so much for your detailed explanations! I see what I was missing: "method forEach returns UNDEFINED after execution". I haven't expected that at all! I am new to JavaScript, and in other languages that I know I have never seen anything like that.
Again, thank you so much for helping me to figure it out, much appreciated!