Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial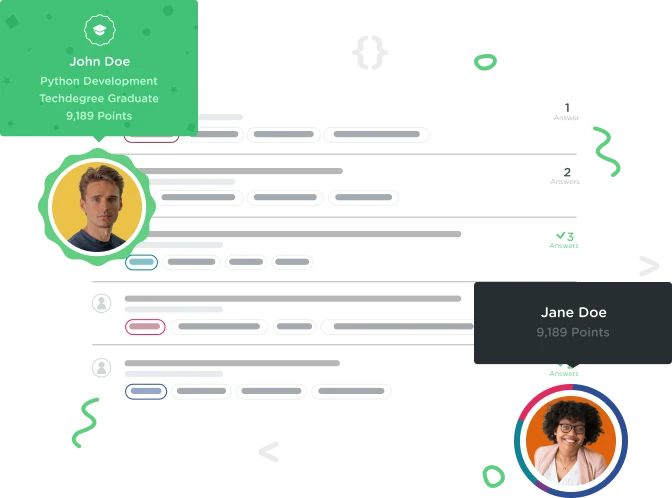

Jennifer Christie
4,038 PointsWhy do I have to define the variable outside of the loop?
function print(message){
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
for(var i = 0; i < students.length; i ++) {
var student = students[i];
var studentList = '<h2>Student: ' + student.name + '</h2>';
studentList += '<p>Track: ' + student.track +'</p>';
studentList += '<p>Points: ' + student.points + '</p>';
studentList += '<p>Achievements: ' + student.achievements + '</p>'
};
print(studentList);
This is my code. I watched the video to see why only the last object in my array appeared, and this was the solution.
var studentList = '';
function print(message){
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
for(var i = 0; i < students.length; i ++) {
var student = students[i];
studentList += '<h2>Student: ' + student.name + '</h2>';
studentList += '<p>Track: ' + student.track +'</p>';
studentList += '<p>Points: ' + student.points + '</p>';
studentList += '<p>Achievements: ' + student.achievements + '</p>'
};
print(studentList);
My question is why do I have to declare the variable first in order for it to loop? Why didn't work when I declared it in the loop?

Jennifer Christie
4,038 PointsThank you for responding Robert.
2 Answers
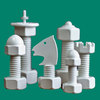
Steven Parker
230,688 PointsDeclaring the variable before the loop allows you to add to it each time through the loop. Then when the loop finishes it will have all the values from every cycle.
If you declare it inside the loop instead, it will start over each time and when the loop is done it will contain only the values from the very last person.

Jennifer Christie
4,038 PointsThanks, Steven.
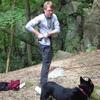
Chadwick Savage
11,919 PointsSo the variable studentList is declared locally in your code in that it only exists inside the loop. It also is reset everytime the loop runs. So if you want to continually add to the list it must be global. Also your print is outside the loop, so the studentlist variable doesn't exist out there. Was it throwing an error that said that studentlist was null or was it printing a variable with only the last student in the list? It should have been saying studentlist was null if I understand correctly.
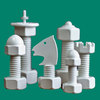
Steven Parker
230,688 PointsVariables declared with "var" are not restricted to block scope. But what you are thinking of would have been an issue if the variable had been declared using "let".

Jennifer Christie
4,038 PointsThere were no errors, it just didn't loop through. It only displayed the last person in the object.
Robert Louis
50 PointsRobert Louis
50 PointsJennifer Christie ,
studentList variable is outside of loop because here we want to concatenate every student details in studentList variable.If you will define studentList variable inside of a loop than every time you loop second time the value of studentList will be set as a empty value.