Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial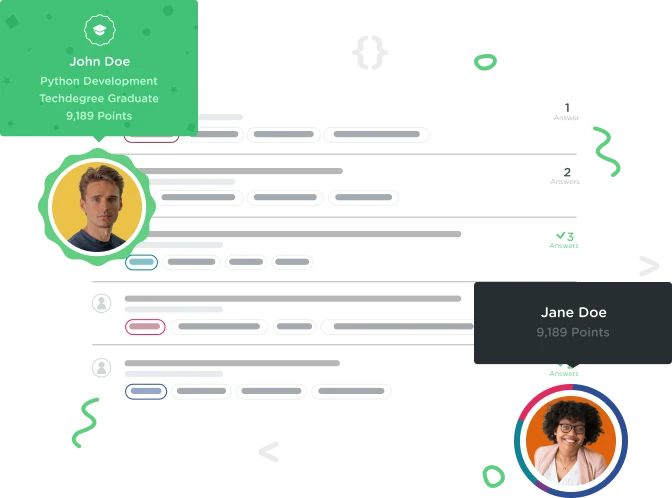
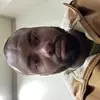
Joshua Moten
2,404 PointsWhy do I have to regenerate console.log(generateNum) in the console in order for it to show?
// Collect input from a user
input = prompt("Enter a number: ");
// Convert the input to a number
convertNum = parseInt(input);
// Use Math.random() and the user's number to generate a random number
generateNum = Math.floor(Math.random() * convertNum) + 1;
// Create a message displaying the random number
if (generateNum < 10) {
document.querySelector('main').innerHTML = `<h2>Check your browser console</h2>`;
console.log(generateNum);
} else if (generateNum >=10 && generateNum < 50){
alert(`Your new generated number: ${generateNum}`);
} else {
document.querySelector('main').innerHTML = `<h2>Your new generated number: <span style='color: gold;'>${generateNum}</span></h2>`;
}
By the way this was my solution for the random number generator but I decided to use all three display methods using some of the techniques I just learned from the previous lessons while using conditionals :)
1 Answer
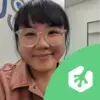
Rachel Johnson
Treehouse TeacherHey Joshua Moten , thanks for your question and for sharing your solution.
You're off to a great start! I like that you even did a bit of extra research to figure out how to deal with NaN
s.
I'm actually not 100% sure about your question, but based on the way the code is written, I'm assuming the following. When entering certain numbers, you get an alert showing the newly generated number, but it does not appear in the console until you enter console.log(generateNum)
in the console.
If so, this is because of the code in your else if
and else
blocks. These blocks run if the generated number is greater than 10. If it's between 10 and 50, we get an alert. If it's greater than 50, we see it displayed on the webpage. We don't have a console.log()
statement in either block, so it won't appear in our console. In these cases, running console.log(generateNum)
in the console will print out that generated number.
I do hope I interpreted your question correctly!
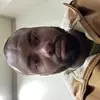
Joshua Moten
2,404 PointsI figured it out lol...the first solution I submitted I forgot to include the console.log after the alert but when I submitted the solution here I had implemented the console.log().
programmer error on why it did not show in the console lol Thank you for the explanation and I am really enjoying the course
Joshua Moten
2,404 PointsJoshua Moten
2,404 PointsI updated the code to check for if it's a NaN...I had to research a little to find what I was looking for