Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial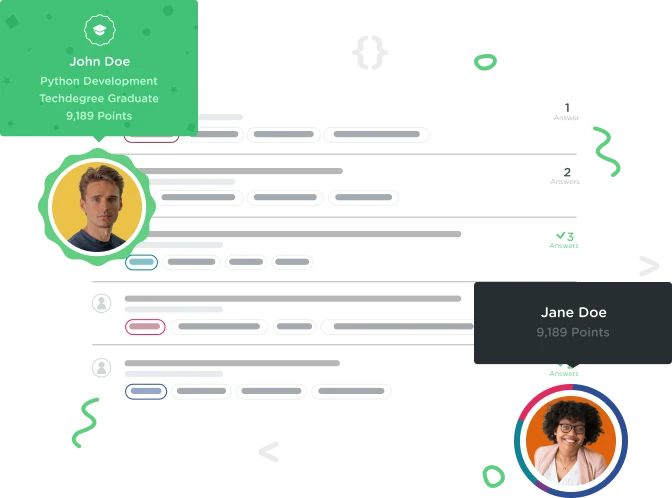

Luke Tate
Courses Plus Student 2,256 PointsWhy do I keep getting a return is outside the function?
For some reason, I keep getting a return is outside a function, even though I did just that, because of indention.
class Book:
def __init__(self, author, title):
self.author = author
self.title = title
def __str__(self):
return f"{self.author}, {self.title}"
def __eq__(self, other):
return self.author == other.author and self.title == other.title
book_a = Book("J.F Rowling", "Harry Potter")
book_b = Book("J.F Rowling", "Harry Potter")
if book_a == book_b:
return True
else:
return False
from book import Book
class BookCase:
def __init__(self):
self.books = []
def add_books(self, book):
self.books.append(book)
1 Answer
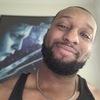
Brandon White
Full Stack JavaScript Techdegree Graduate 35,763 PointsHi Luke Tate,
The return keyword is used to return a value from, and exit a function.
You got an if...else statement where you return True and/or False, but the if...else statement is not nested within a function. That's why you're being told that return is outside the function.
In book.py you can delete all the code not in the Book class definition. Your str, and eq methods are perfect.
But if you just want to evaluate the expression book_a == book_b, there's no need to make it the test of an if...else expression, just to get a True or False value. The expression already evaluates to a boolean.
Just like you wouldn't need to write something like:
if 5 + 5 == 10:
return 10
You can just leave it book_a == book_b
Luke Tate
Courses Plus Student 2,256 PointsLuke Tate
Courses Plus Student 2,256 PointsThanks for your advice! I will keep that in mind. This was for the second challenge, and oddly enough I just needed to just have an dunder comparison method.