Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial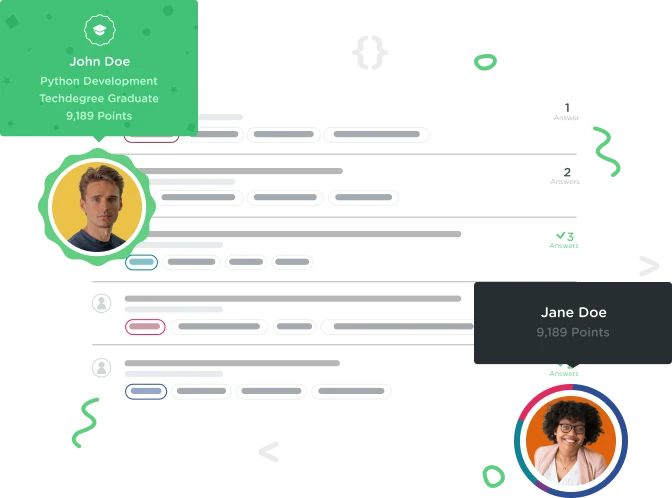

Sean Ball
10,501 PointsWhy do I keep getting a type error of undefined on my prompt when I use the [i][0] index?
See my code below. When I run this, I get a type error of undefined on line 12 at the [0], I do not understand what I am doing incorrectly here.
const QA = [
['sharks have', 'teeth'],
['ghosts have', 'ectoplasm'],
['skinwalkers have', 'no friends']
];
let correct = 0;
const question = (arr) => {
for (let i = 0; i <= arr[0][1].length; i++) {
q = prompt(`answer the following question: ${arr[i][0]}`)
if (q === arr[i][1]) {
parseInt(correct++)
}
}
}
question(QA)
document.querySelector('main').innerHTML = `
<ol>
${question(QA)}
You answered ${correct} correctly
</ol>
`;
Mod edit: added forum markdown for code. Check out the "markdown cheatsheet" linked below the comment box to for info on wrapping code blocks.
1 Answer
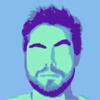
Cameron Childres
11,818 PointsHey Sean,
It's the condition in your for loop that's causing the problem:
i <= arr[0][1].length
arr is accessing your QA array. The 0 index item is another array and the 1 index item in that array is the string "teeth". The length of "teeth" is 5.
i starts at 0 and you're having the loop run as long as i is less than or equal to 5, meaning it will try to run 6 times:
Loop 1: i=0 and arr[i][0] = "sharks have"
Loop 2: i=1 and arr[i][0] = "ghosts have"
Loop 3: i=2 and arr[i][0] = "skinwalkers have"
Loop 4: i=3 and arr[i][0] = undefined
Loop 5: i=4 and arr[i][0] = undefined
Loop 6: i=5 and arr[i][0] = undefined
When it tries to run loop #4 and hits undefined it gives up and throws the error. You can fix this by rewriting the condition to take just the length of the QA array and run as long as i is less than that length. This will loop 3 times.
i < arr.length
One more thing to mention -- you've got question(QA)
twice in your code: once on its own and again in the template literal. This will make it run two times and return "undefined" inside your template literal.
I hope this helps! Let me know if you have any questions.
Sean Ball
10,501 PointsSean Ball
10,501 PointsThank you so much!