Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial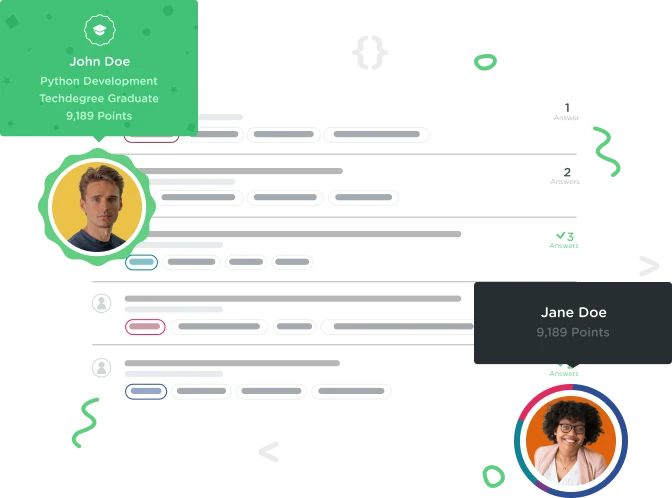

Susan Rusie
10,671 PointsWhy do I keep getting this error?
$ node app.js
C:\Users\Rusie\Documents\susan_treehouse\Node.js_Basics_2017\Getting_the_Respons
e_Body\Stage_2_Video_4_0_start\app.js:16
let body = "";
^^^
SyntaxError: Block-scoped declarations (let, const, function, class) not yet sup
ported outside strict mode
at exports.runInThisContext (vm.js:53:16)
at Module._compile (module.js:373:25)
at Object.Module._extensions..js (module.js:416:10)
at Module.load (module.js:343:32)
at Function.Module._load (module.js:300:12)
at Function.Module.runMain (module.js:441:10)
at startup (node.js:139:18)
at node.js:968:3
I have looked over this code and can't find my mistake. What am I missing? Any help on this is greatly appreciated. Please and thank you in advance.
Here is my code:
// Problem: We need a simple way to look at a user's badge count and JavaScript points
// Solution: Use Node.js to connect to Treehouse's API to get profile information to print outerHTML
// Require https module
const https = require('https');
const username = "chalkers";
//Function to print message to console
function printMessage(username, badgeCount, points) {
const message = '${username} has ${badgeCount} total badge(s) and ${points} points in JavaScript';
console.log(message);
}
// Connect to the API URL (https://teamtreehouse.com/username.json)
const request = https.get(`https://teamtreehouse.com/${username}.json`, response => {
let body = "";
//Read the data
response.on('data', data => {
body += data.toString();
});
response.on('end', () => {
// Parse the data
console.log(body);
console.log(typeof body);
// Print the data
});
});
```
2 Answers

Kenya Sullivan
21,711 PointsIt depends on your version of node.js, the latest version of node no longer requires strict mode for ES6. I didn't have the issue. http://node.green/ shows what is supported.
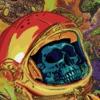
kramer
3,515 PointsTry adding "use strict"; at the beginning of the file

Susan Rusie
10,671 PointsThank you. That was what was needed to make the program work the way it should. I wish they would have mentioned that in the video, though.