Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial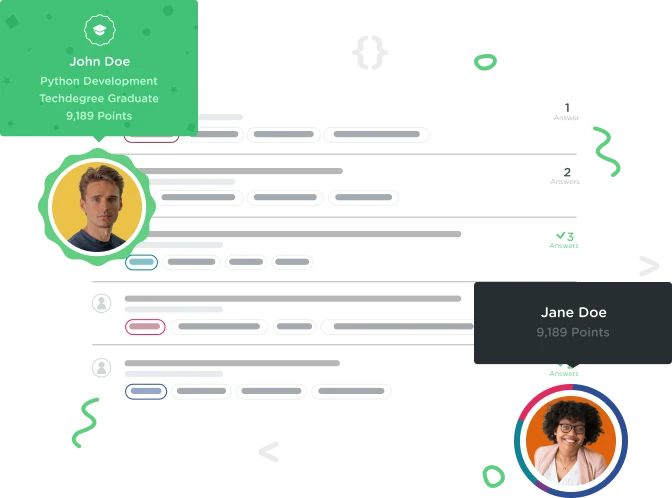

Ryan Broughan
12,742 PointsWhy do I need different variable names when using separate .js files?
I solved this challenge by creating a separate .js file to hold my rooms.json ajax query.
Initially, ONLY the Meeting Rooms part worked (it was linked second in the html). Once I gave each ajax request unique variables for the XMLHttpRequest objects I regained functionality in the Employee Office Status tool.
It seemed to me if the .js files are different the variables shouldn't clash. What am I missing?
Also, is linking to 2 distinct .js files strictly worse than using 1 file to hold both queries?
2 Answers
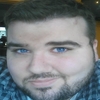
Marcus Parsons
15,719 PointsHey Ryan,
One of the great things about JavaScript is the availability of variables/objects/functions across scripts (and also a downfall). Every variable that you declare goes into the global scope and is available to all other scripts except for variables declared with var
keyword inside a function, which are local only to that function.
If you were to wrap each section of code into a function, you will see the conflict go away, because the xhr variable will only belong to the function, not to the global scope, and no conflicts will arise. Try this out:
function getEmployeesData () {
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if(xhr.readyState === 4) {
var employees = JSON.parse(xhr.responseText);
var statusHTML = '<ul class="bulleted">';
for (var i=0; i<employees.length; i += 1) {
if (employees[i].inoffice === true) {
statusHTML += '<li class="in">';
} else {
statusHTML += '<li class="out">';
}
statusHTML += employees[i].name;
statusHTML += '</li>';
}
statusHTML += '</ul>';
document.getElementById('employeeList').innerHTML = statusHTML;
}
};
xhr.open('GET', '../data/employees.json');
xhr.send();
}
function getRoomsData () {
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if(xhr.readyState === 4) {
var rooms = JSON.parse(xhr.responseText);
var statusHTML = '<ul class="rooms">';
for (var i=0; i<rooms.length; i += 1) {
if (rooms[i].available === true) {
statusHTML += '<li class="empty">';
} else {
statusHTML += '<li class="full">';
}
statusHTML += rooms[i].room;
statusHTML += '</li>';
}
statusHTML += '</ul>';
document.getElementById('roomList').innerHTML = statusHTML;
}
};
xhr.open('GET', '../data/rooms.json');
xhr.send();
}
getEmployeesData();
getRoomsData();
There are a number of ways that you can wrap the code into a function, but I did it the most easily legible way, in my opinion.

Paco Bahena
Courses Plus Student 5,857 PointsRemember that you could declare variables within your functions and make them private instead of global. But, maybe for your problem you needed a global scope.
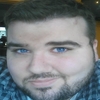
Marcus Parsons
15,719 PointsPutting these variables into the global scope is the problem.
Ryan Broughan
12,742 PointsRyan Broughan
12,742 PointsI guess I never really understood how global the global scope really is. Hah.
Also, I really like this solution--it not only fixes my issue, but makes the code reusable as well. Very insightful.
Thanks Marcus!
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsYou're very welcome, Ryan! Whenever you make a variable outside of a function (or don't attach the
var
keyword to a never before used variable inside of a function), it attaches itself to thewindow
object, which is the global-est object (if that's even a real word lol). A better way to say that is that it is the root object of everything. When it does that, everything that is loaded (or can be loaded) into that window will have access to it. And when I say variable, I mean it in the broad sense of objects/arrays/element references/everything lol. It also means that you can writewindow.variablename
instead of justvariablename
but there's no point to that haha All browsers know that when you write JavaScript, you are writing in thewindow
object, so there's no need to reference it.Did you have any more questions?
Giuseppe Ardito
14,130 PointsGiuseppe Ardito
14,130 PointsGreat stuff!