Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial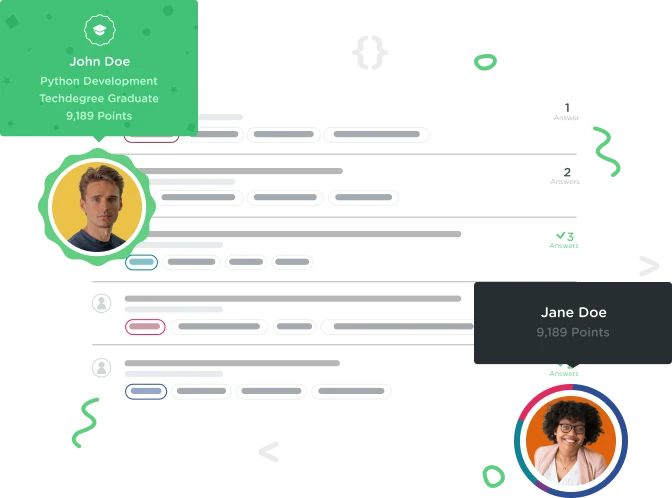

Samar Khanna
1,757 PointsWhy do i need to pass in two arguments in the init method when that was already being done earlier?
It makes no sense. I was already feeding in arguments for firstName and lastName before the init method was added. Why did i have to do that all over again when I created the init method?
Also, when i created the property called type, why wasn't the system asking me to feed in a value for the property after the init method was created?
1 Answer
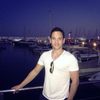
Jayden Spring
8,625 PointsWhen you have custom initialisers in Struct's they take arguments. Say you had an arguments firstName & lastName and you have two constants in the struct firstName and lastName.
struct Names{
let firstName : String
let lastName : String
var age : Int?
init(firstName: String, lastName: String)
{
//firstName = firstName Wouldn't work as the compiler would think you are talking about firstName within scope
self.firstName = firstName //Indicates you want to access a variable outside scope and assign it the one from within
self.lastName = lastName
}
}
Whenever you create an instance of the struct you need to pass it the values that you specify in the custom initialiser. A struct is different to a class which you will learn further down the course, but the idea is when you initialise it you will assign it to a variable and then do you're work from there. eg:
var names = Names(firstName: "John",lastName: "Doe")
names.age = 28
println("\(names.firstName) \(names.lastName) is \(names.age!)")
As you can see from that block, since we init'd the struct and assigned it to 'names' we can then manipulate the optional variables within the struct or access any other methods within it.
Hope this helps.
Samar Khanna
1,757 PointsSamar Khanna
1,757 PointsAlso what was the point of saying self.firstName in the init method? My code worked fine without self