Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial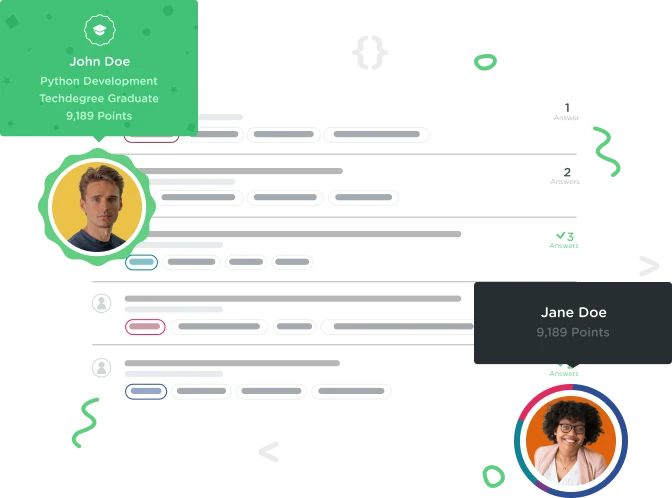

Benjamin Thurston
2,720 PointsWhy do this keep erroring out? I have tried multiple ways of working this code and nothing has worked.
--C#
string input = Console.ReadLine();
int temperature = int.Parse(input);
if(temperature <= 21);
{
Console.WriteLine("Too cold!");
}
else if(temperature <= 22)
{
Console.WriteLine("Just right.");
}
else(temperature >= 22)
{
Console.WriteLine("Too hot!");
}--
string input = Console.ReadLine();
int temperature = int.Parse(input);
if(temperature <= 21);
{
Console.WriteLine("Too cold!");
}
else if(temperature <= 22)
{
Console.WriteLine("Just right.");
}
else(temperature >= 22)
{
Console.WriteLine("Too hot!");
}
3 Answers
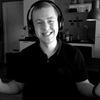
Rune Andreas Nielsen
5,354 PointsHi, Benjamin.
Your first error is here on line 3. "if(temperature <= 21);"
if(temperature <= 21);
The error is the ';' semicolon. C# throws an error if you use a semicolon in a conditional statement.
Your second error on line 11.
else(temperature >= 22)
You cannot set a condition on an 'else' statement because the 'else' keyword is used when no other of your conditions has been met, and therefore shouldn't use a conditional.
The final result should be. I cannot remember the exercise, but if you need to test on "temperature >= 22" in the last statement, you should use an 'if else'.
string input = Console.ReadLine();
int temperature = int.Parse(input);
if (temperature <= 21)
{
Console.WriteLine("Too cold!");
}
else if (temperature <= 22)
{
Console.WriteLine("Just right.");
}
else
{
Console.WriteLine("Too hot!");
}
Good luck, and happy programming! :)
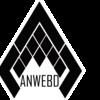
Angyal István
11,259 PointsYou should not write the third statement, cause You write before "else" and not "else if", in that case try this:
if(temperature <= 21);
{
Console.WriteLine("Too cold!");
}
else if(temperature <= 22 && temperature > 21) // You should use logical AND to specify it more.
{
Console.WriteLine("Just right.");
}
else
{
Console.WriteLine("Too hot!");
}
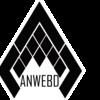
Angyal István
11,259 PointsAnd in Your code take care for that:
else if(temperature <= 22) { Console.WriteLine("Just right."); } else(temperature >= 22) { Console.WriteLine("Too hot!"); }
In that case the value 22 is can be applied both statement, at now just that switch wich comes first.

Benjamin Thurston
2,720 PointsI had an ID10T error. Thanks I just had to take out the = sign