Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial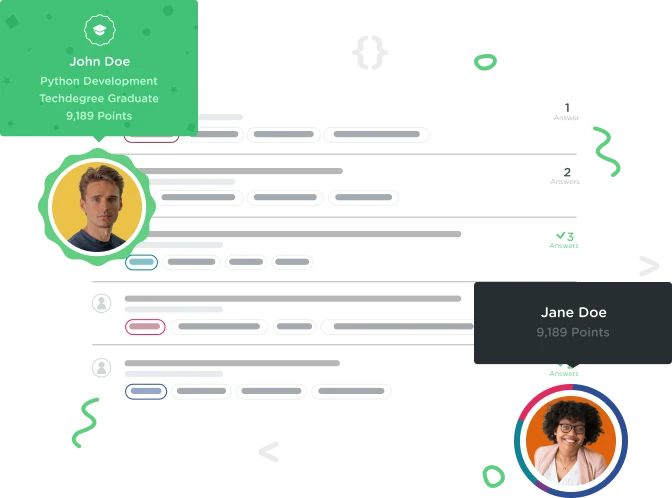

Walker Bolger
16,798 PointsWhy do we call the functions without parentheses i.e. '()'?
We created two functions: passwordEvent() and confirmPasswordEvent(). Normally when you call a function, you have to include parentheses like we did in an earlier course when we created a random number alert.
function alertRandom() { var randomNumber = Math.floor( Math.random() * 6 ) + 1; alert(randomNumber); }
alertRandom(); //notice the parentheses after 'alertRandom'
Yet when we call the functions in '$($password).focus(passwordEvent).etc' we do not put the parentheses after the functions we call. Why is that? Shouldn't the last lines of code look like '$($password).focus(passwordEvent()).etc'?
2 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Walker,
Without parentheses you're not actually calling the function. A function name without the parentheses is a reference to the function.
We don't use the parentheses in that code because we don't want the function to be called at the point where that code is encountered. Instead, we want to pass a reference to our function into the .focus()
method and that method will call our function for us whenever the element receive focus.
With this code $($password).focus(passwordEvent())
what would happen is that the passwordEvent function would be called immediately and the return value of that function is what would get passed into the focus method. This doesn't work because the focus method is expecting a function to be passed in.

Walker Bolger
16,798 PointsI think I understand now. It's because the focus handler requires a function. This is similar to why when we call function .hasClass() we don't pass in the '.' when we pass in the class, correct?

Jason Anello
Courses Plus Student 94,610 PointsIf I understand correctly, you're talking about the '.' we put in front of class names when writing class selectors?
I wouldn't say this is similar to your function question. This is more of a syntax issue.
When writing css class selectors in a css file a dot in front is required as part of the syntax. Same thing with css selectors written in jQuery like $('.intro')
The dot is required because it's a selector.
With the jQuery hasClass()
method you need to simply pass in the class name. You're not passing in a class selector but just the class name.
Let me know if I misunderstood your question.
Timothy Bramlett
6,609 PointsTimothy Bramlett
6,609 PointsThanks, this is still confusing me a bit, but I think I have encountered it before.
We obviously don't want the result of the function
passwordEvent()
, which doesn't even have a return value, but we want the reference to it so that.focus()
can use it as it wants to use it. Does that sound correct?edit: After thinking about this for a few minutes, I realized I have encountered this before in vanilla JavaScript when binding event handlers with functions (I think that is the correct way to describe it).
Basically I told it what function to use when an event happened. When I did this I could only use
.functionIWantToRun
and not.functionIWantToRun()
.Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsHi Timothy,
That's correct.
Your example of event binding is a common use case for passing in function names without parentheses and that's really what we're doing in this code except with jQuery and not plain vanilla javascript.
When you have
.focus(passwordEvent)
you're binding thepasswordEvent
handler to the focus event. This is a shortcut for.on('focus', passwordEvent)
From the jQuery docs, https://api.jquery.com/focus/
For another example that's not related to event handling you can take a look at the sort method. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/sort
The sort method can take an optional compare function for an argument.
Example:
Notice that when I passed in the
compareNums
function as an argument to thesort
method I didn't put parentheses after the function name. This is because I don't want the function to be called right then and there but rather I want the sort method to have a reference to this compare function so that it can call it as needed while it's trying to sort the array.This is very similar to the event handling code for this project.
I hope this helps clear it up for you.