Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial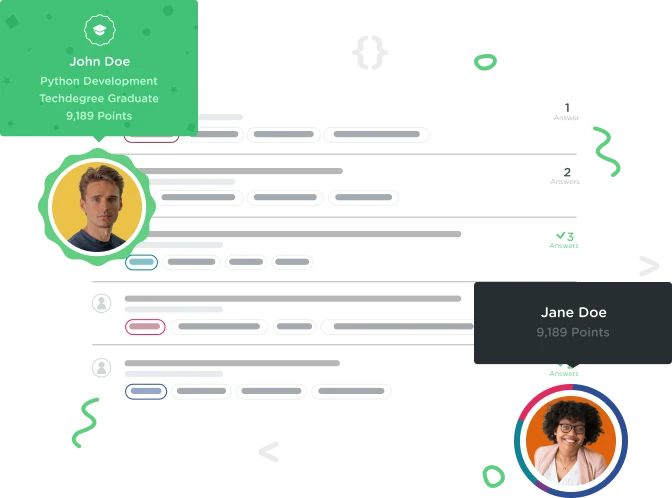

shu Chan
2,951 PointsWhy do we have () after isEmpty() ?
Why do we have () after isEmpty() ? What's it for? why do we need it? why doesn't Johnny Depp have an Oscar yet?
3 Answers
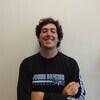
Charles Kenney
15,604 PointsBecause you are calling a method of the String class. Just like any other function or method, it is invoked with a set of parentheses, empty or otherwise.
If you wanted to understand more in depth how this method works, basically: it returns true if the strings length evaluates to 0. Very simple. This is how its source code looks.
public final class String {
public boolean isEmpty() {
return count == 0;
}
}
So when you call the isEmpty method on a given string, the count property (which counts the number of characters) is compared with zero, and a boolean is returned describing that.
You can find more on the source code of Java's primitive types / objects here.
Hope this helps -Charles

shu Chan
2,951 PointsThank you for your answer,
if I am clear about this than, the () are for invoking a function of an object, and this is necessary because if JAVA did not have () than it would not know what to do with the function sayHelloWorld because all I have done in instance.sayHelloWorld is mention it. Is that correct?
Also from you explanation you say that in order to make use of a function we must create an instance of the class it belongs to. Is this because this is encapsulated on a second file? What if I created the class on the Main.Java file? would I still need to create an instance of the class even though it belongs on the same file?
Do I have to create a new file for each new class I create?
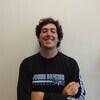
Charles Kenney
15,604 PointsYes you are completely correct about invoking the function. Glad to see you understand that now.
As far as classes go, don't stress out about learning them in depth before you go over them in the Treehouse course. But it is good that you are curious. I will try to answer your question without getting too much in detail.
It is conventional to put classes in separate files. This helps to organize your code base. To use a class declared in a separate file, you make it public (or accessible to other files/modules) using the public access-modifier. This is done simply by prepending your class with the keyword 'public'.
Now more on classes themselves: you can think of a class as a model, or a blueprint that represents a type but isn't the type itself. For instance, the String class represents the type of String and provides methods and properties that we can use to manipulate strings in our code, but the class itself is not a usable String, it is a blueprint for a string.
You instantiate (or create instances of) classes with the following syntax:
// CLASSNAME NAME = new CLASSNAME(PARAMETERS)
String myString = new String("Hello, World")
String myOtherString = "Hello World"
// String => Class
// myString => "Hello World"
If this seems confusing at all, do not worry. It may take you a while to get used to object oriented programming. Keep practicing, but don't stress out about not understanding this. It will come with time
-Charles

shu Chan
2,951 PointsThank you Charles, your explanation was wonderful!
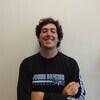
Charles Kenney
15,604 PointsNo problem, enjoy learning!
shu Chan
2,951 Pointsshu Chan
2,951 PointsHey Charles thanks for the answer, but I am still rather confused. Could you break down what you mean by "Because you are calling a method of the String class"?
greatly appreciate your time and effort
Charles Kenney
15,604 PointsCharles Kenney
15,604 PointsNo problem,
If the word method is confusing you, think of it as a function which belongs to an object/class.
Consider the following code
This may or may not seem confusing at first, so bare with me.
In this example, we have created a class called myClass. This class has one method with a return type of void (nothing) and it's name is sayHelloWorld. It's functionality it simple: it logs the string "Hello World" to the console.
In order to make use of this functionality, we must create an instance of myClass. We have done so in the Main.java file and assigned the instance literally, to the name 'instance'. On this instance, we can use dot notation to access the method sayHelloWorld that we previously defined. Just as with a function, if we append a set of parentheses, we can invoke it (or call, or execute). This simply acts as a pointer to our previously defined function block, which looks like this:
System.out.println("Hello World");
So when it is invoked, that block of code is executed by the processor just as if we wrote it ourself in the global context.
What you're doing with the string class is not any different. When you initialize a string, you are just using a simplified syntax of what we have just done, using string literal (a literal implementation of a string, eg "Hello World").
String myString = "Hello World";
translates to:
String myString = new String("Hello, World");
Now that you understand that. Simply imagine that, just as we have created a method for the 'myClass' class earlier, the core developers of Java implemented an isEmpty method for the 'String' class. When you use dot notation to get the computed property 'isEqual' you are really executing a function that evaluates whether or not the strings length is equal to zero, just as stated in the previous answer. And the result of that function is a True/False boolean; unlike our implementation which has no return value.
You can play around with methods here.
Does this clear everything up?
-Charles