Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial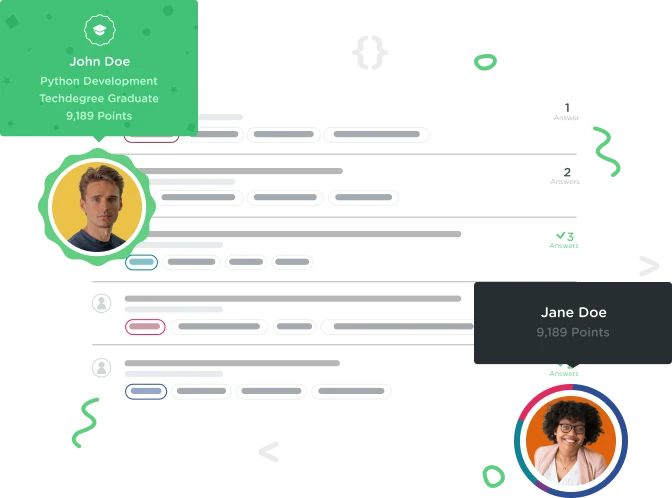

Adam Mata
3,984 PointsWhy do we have to specify > -1?
My initial question was why my program was returning 'apples' as ' is not in stock' when it is clearly in the array.
var inStock = [ 'apples', 'eggs', 'milk', 'cookies', 'cheese', 'bread', 'lettuce', 'carrot', 'broccoli', 'pizza', 'potato', 'crackers', 'onion', 'tofu', 'frozen dinner', 'cucumber'];
var search;
function print(message) {
document.write( '<p>' + message + '</p>');
}
while ( true ) {
search = prompt("Search for a product in our store. Type 'list' to show all of the produce and 'quit' to exit");
search = search.toLowerCase();
if ( search === 'quit' ) {
break;
} else if ( search === 'list' ) {
print( inStock.join(', '));
} else {
if (inStock.indexOf( search ) ) {
print( 'Yes, we have ' + search + ' in the store.' );
} else {
print( search + ' is not in stock.' );
}
}
}
I went back and realized I had not added the '> -1' after my indexOf method and as soon as I had, my code worked as it should. After getting it to work that specific if statement now looks like this
if ( inStock.indexOf( search ) > -1 ) {
print( 'Yes, we have ' + search + ' in the store.' );
}
I just want to know why is it that without that simple 'less than -1' the 0 item in the array showed as 'not in stock' or followed the path as if it wasn't in the inStock array.
2 Answers

Alexander La Bianca
15,959 Points2 things are going on here.
The array.indexOf method returns -1 if the item is not in the array. And returns the index of the item in the array. If the item is larger than -1 then you enter the truthy if block. (because an index of an array is always 0 or larger)
The reason why it did not work in your case is because 'apples' is at index 0....now 0 inside a conditional evlauates to false. so if(false) will never go into the if block. Which is why it went inside the else.... It would have worked if you tried to do 'eggs' as that is at index 1 and 1 always evaluates to true inside a conditional.
it evaluates to the following when you don't include the >-1
if(0) {} //in the case where you search for apple this is the same as
if(false) {} //will never be true
//now if you tried for eggs it would have been
if(1) {} //which evaluates to
if(true) {}
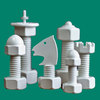
Steven Parker
231,269 PointsWithout the comparison, the "if" was converting the result of the "indexOf" call directly into a boolean. Since "apples" is the first item it has an index number of 0. And since 0 is "falsey" it considered it to be "not in stock".
Anything else, including things that don't exist, would appear to be in the store.