Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial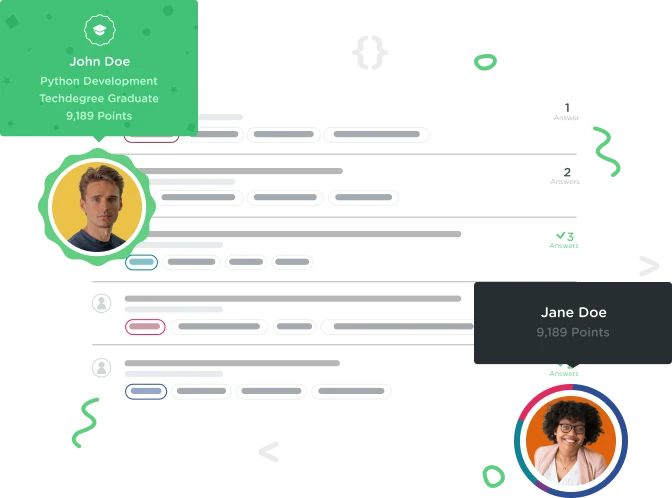

Hasan Rayan
Courses Plus Student 91 PointsWhy do we need a Map, if we have Object?
With an object, I can do the following: let marks = {math: 40, physics: 50, chemistry: 45}
I can set, get, find, delete and clear. Then, why we need a Map?
In the documentation, it says: "The Map object holds key-value pairs. Any value (both objects and primitive values) may be used as either a key or a value."
Also, the Object can holds key-value pairs. And, any value may be used as either a key or a value. So again, why we need a Map?
2 Answers
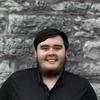
Michael Hulet
47,912 PointsThe MDN Documentation has a pretty great comparison on this. For those who don't want to click through the link:
Objects
are similar toMaps
in that both let you set keys to values, retrieve those values, delete keys, and detect whether something is stored at a key. Because of this (and because there were no built-in alternatives),Object
s have been used asMaps
historically; however, there are important differences that make using aMap
preferable in certain cases:
- An
Object
has a prototype, so there are default keys in the map that could collide with your keys if you're not careful. As of ES5 can be bypassed by usingmap = Object.create(null)
, but is seldom done.- The keys of an
Object
areStrings
andSymbols
, whereas they can be any value for aMap
, including functions, objects, and any primitive.- You can get the size of a
Map
easily with thesize
property, while the size of anObject
must be determined manually.This does not mean you should use
Maps
everywhere. If you're not sure which one to use, ask yourself the following questions:
- Are keys usually unknown until run time? Do you need to look them up dynamically?
- Do all values have the same type? Can they be used interchangeably?
- Do you need keys that aren't strings?
- Are key-value pairs frequently added or removed?
- Do you have an arbitrary (easily changing) number of key-value pairs?
- Is the collection iterated?
If you answered 'yes' to any of those questions, that is a sign that you might want to use a
Map
. Contrariwise, if you have a fixed number of keys, operate on them individually, or distinguish between their usage, then you probably want to use anObject
.
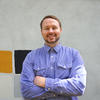
Tom Geraghty
24,174 PointsSpeed.
To add on to the excellent answer from Michael Hulet, I also wanted to state there are performance reasons why you use Map over other (potentially more primitive) collection types.
https://jsperf.com/es6-map-vs-object-properties/99
There are probably better resources, but that jsperf link shows that iterating over Map is almost always faster in almost every browser.
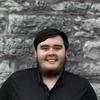
Michael Hulet
47,912 PointsI know that Map
s are designed to be faster, but an interesting note is that according to that JSPerf, Object
is twice as fast in Safari 11.0 (13604.1.38.1.6) than it is in Chrome 61.0.3163.100 on my mid-2015 MacBook Pro (2.2 GHz Intel Core i7 processor, 16 GB 1600 MHz DDR3 RAM) running the last developer beta of macOS 10.13.0 High Sierra (17A360a)