Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial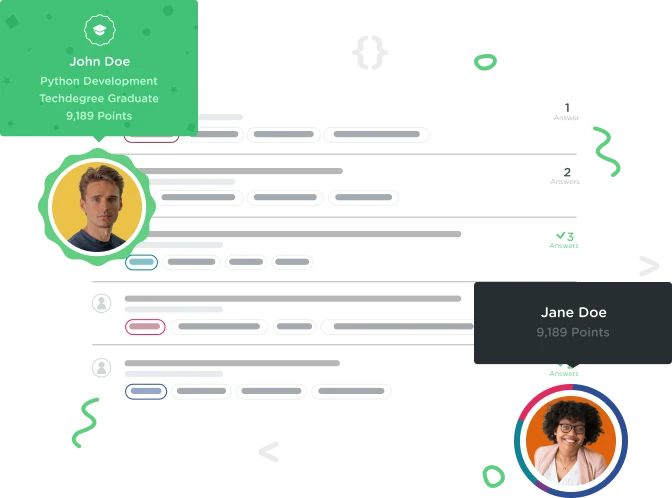

vamosrope14
14,932 PointsWhy do we need an if statement for the status code if we are already applying a try and catch block to the code?
.
2 Answers
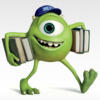
Tobias Edwards
14,458 PointsBefore the if-statement for the status code, we have:
const getProfile = username => {
try {
https
.get(`https://teamtreehouse.com/${username}.json`, response => {
let body = ''
response.on('data', data => {
body += data.toString()
})
response.on('end', () => {
try {
const profile = JSON.parse(body)
console.log(
printMessage(
profile.profile_name,
profile.badges.length,
profile.points.JavaScript
)
)
} catch (e) {
console.error('Invalid username')
}
})
})
.on('error', e => {
printError(e)
})
} catch (e) {
printError(e)
}
}
- The first try-catch block will catch errors in visiting the URL e.g. problems with the URL
- The second try-catch block within the
response.on
block will catch errors thrown byJSON.parse()
Why have an if-else condition to check for status codes? e.g. suppose we enter a valid URL, and pass a valid username. But, the treehouse website is down, and so will throw a 500 error! Our code will catch the error and say the problem is that the data couldn't be parsed. This is wrong - the actual error that should have been outputted is "500 error".
I had the same question as you, and this is what I thought of? Please correct me if I'm wrong.
TLDR; The if-else condition is needed to catch and display the appropriate error message.

Henry Blandon
Full Stack JavaScript Techdegree Graduate 21,521 PointsTobias Edwards, I agree for the most part, I had the same question. I agree you need different try/catch for each specific task. However, if you get any status code deficient than 200 the code that parse the response wont run. I think a better example would be: if the status code is a 200, good response, but the server sends no data at all, or some random things not in Jason format, then the try/catch for the JSON.parse would run and catch the error. This is just an opinion base on how I understood, I am just an student.