Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial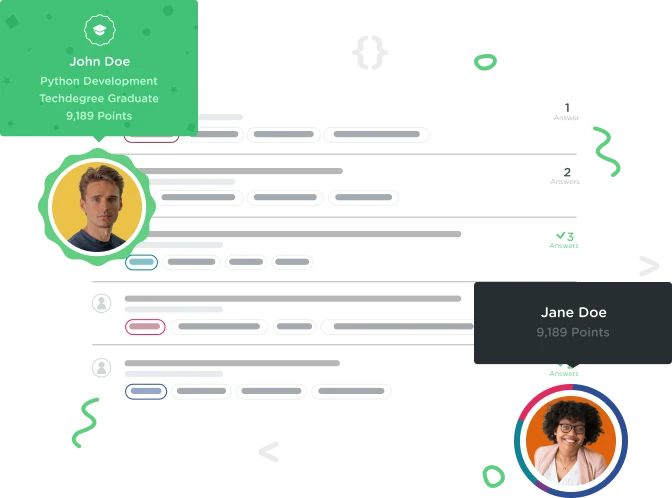
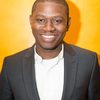
Welby Obeng
20,340 Pointswhy do we need self in class method?
Take a look at the code below
class Monster:
hit_points = 1
color = 'yellow'
weapon = 'sword'
sound = 'roar'
def battlecry(self):
return self.sound.upper()
Why do we need self in the method..why cant we just do
def battlecry() return sound.upper()
?
5 Answers
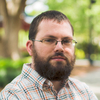
Kenneth Love
Treehouse Guest Teacherself
is the current object. sound
doesn't exist. It either belongs to the class, as Monster.sound
or to the instance as self.sound
. Since we don't want to refer to the class version of it (what if we changed the value in our instance?), we have to use self
. Since the method also applies to the instance, it has to know what instance to use for looking up values, so it gets a self
argument.

Jegnesh Gehlot
5,452 PointsHere is another explanation to your question:
class Monster: #class defination
hit_points = 1
color = 'yellow'
weapon = 'sword'
sound = 'roar'
def battlecry(self):
sound = 'tweet'
return sound.upper()
jubjub = Monster() #We are defining a monster called jubjub which is instance of class Monster and has a property battlecry.
Expression 1
jubjub.battlecry() #This will give us "tweet"
Expression 2
Monster.battlecry(jubjub) #This will give you the exact same output but that's how python is interpreting Expression 1. If you look at this expression carefully you can see the keyword self is actually the object jubjub. Also, the class attribute sound (which has value "roar") is different than the method variable (which has value "tweet"). The class attribute can be accessed using the key value self (for example self.sound) and is global to the class.
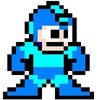
Robert Richey
Courses Plus Student 16,352 PointsHi Welby,
The selected answer from this stackoverflow thread helped me with understanding this same issue.
Best Regards
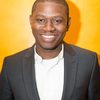
Welby Obeng
20,340 PointsIm still a little confused...:(
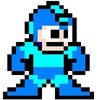
Robert Richey
Courses Plus Student 16,352 PointsAh, sorry. Basically, it was easier for them to implement explicit self and by doing so, there is less computational overhead.
So, stated by the BDFL himself, the only real reason he decided to use explicit self over implicit self is that:
it is explicit
it is easier to implement, since the lookup must be done at runtime(and not at compile time like other languages) and having implicit self could have increased the complexity(and thus cost) of the lookups.
William Li
Courses Plus Student 26,868 PointsThen maybe give this article a try?
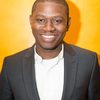
Welby Obeng
20,340 PointsIm still a little confused...:(
Does it have to do with local and global variable?
If I do
class Monster:
hit_points = 1
color = 'yellow'
weapon = 'sword'
sound = 'roar'
def battlecry():
sound = 'tweet'
return sound.upper()
I get x.battlecry() = TWEET but if I add self I get x.battlecry() = ROAR

shezazr
8,275 Pointsself just means that you want to work on the current instance of an object... otherwise you would be changing/using the object directly.. (i could be wrong!)