Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial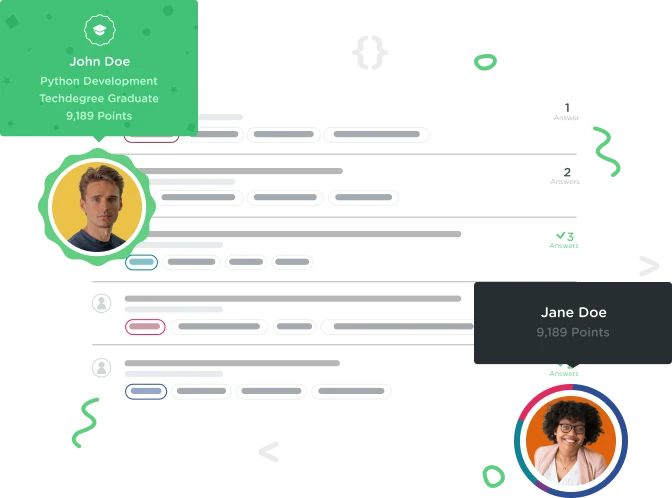

omarshehab
477 PointsWhy do we need to create a listener ?, we tied its method with the "showFactButton" already.
Can someones please explain why a listener is needed sense we already typed the dot operator and accessed the "setOnClickListener()" method shouldn't it be enough for the listener to be working ?
1 Answer

Eran Mani
3,751 PointsHi omar,
you can link the button and the listener with two methods, you can choose which one you like the best:
- Working with anonymous listener - the syntax is this -
findViewById(R.id.button).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { //write your code here... } });
- The second is the one that i prefer the most, since its more "clean" way. you need to implement the onClickListener so the activity itself will use it for you. it is more simple ofcourse. all you have to do is write this code -
findViewById(R.id.button).setOnClickListener(this);
then you will see an error. thats fine, all you have to do is to put your cursor on the line of code and press - alt + enter you will see two options - choose the second one - Make main activity implement android.view.onClickListener, and then a new method will be created for you. this is how it will looks like:
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//you can initialize the button like this
//notice that i find the Button only by its ID that was given in the XML file
findViewById(R.id.button).setOnClickListener(this);
}
@Override
public void onClick(View v) {
//here you will write the code
}
}
hope it helps!
Farouk Charkas
1,957 PointsFarouk Charkas
1,957 PointsThe setOnClickListener is just a bridge between the button and the actual OnClickListener; this is a convenient way to do this as:
showFactButton.setOnClickListener(new OnClickListener);