Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial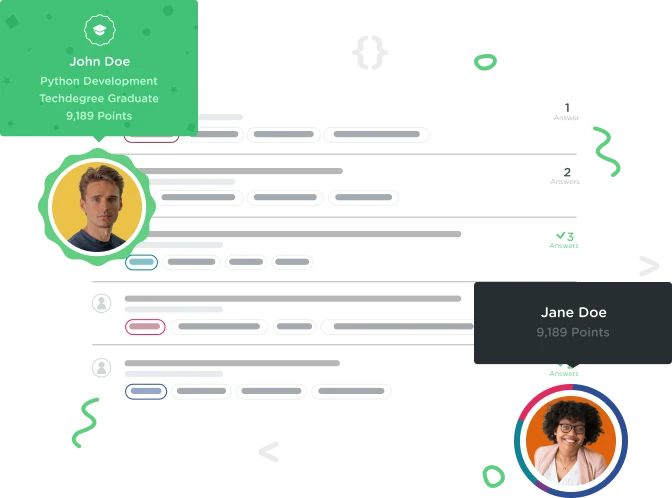
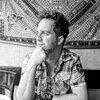
jlampstack
23,932 PointsWhy do we need to declare javascript variables?
In javascript we can use a variable without declaring it, so I am wondering why do we need to even declare it at all?
E.g.) x = 'foo'; // returns foo
What is the importance of using the keyword var?
2 Answers
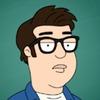
Unsubscribed User
15,444 PointsWhat is the importance of using the var keyword?... Scope.
There are two different levels of scope within javascript, local and global.
Local Scope
When variables are declared within a function, they become local to that function. This means they can't be accessed from outside the function. For example:
// code here can NOT use the foo variable
function myFunction() {
var foo = "bar";
// code here CAN use the foo variable
}
Global Scope
When variables are declared outside a function, they become global. This means that all scripts and functions on a web page can access that variable.
var foo = "bar";
// code here CAN use the foo variable
function myFunction() {
// code here CAN use the foo variable
}
Undeclared variables
When you assign values to variables that haven't been declared, then they will automatically become global variables. So regardless whether or not the variable was assigned a value inside or outside a function, the variable will be global and can be accessed across all scripts and functions on a web page.
myFunction();
// code here CAN use foo variable
function myFunction() {
foo = "bar";
}
Conclusion
When it is said a variable is accessible, it is meant that it is visible and may possibly be overwritten elsewhere on a webpage. So to ensure that the variable is only accessible within the scope you desire, then you need to ensure that you are defining it, either with var keyword, or more practically with the const and let keywords.
Hope this helps,
Regards,
Berian
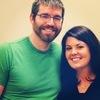
Carson Black
9,320 PointsHello jaycode! I'm still a bit of a beginner, but I believe the main importance is for human readability. If you declare a variable without the 'var' keyword, it may appear to belong to an already declared variable.
E.g.) x = 'foo'; vs. var x = 'foo'; x = 'fum'; x = 'fum';
In the first example, the human reader may believe that the first 'x' is changing a previously declared 'x' variable to 'foo', which is not the case, as there was no previously declared 'x' variable. However, the second example clearly shows that declaring 'x' with a value of 'foo' is the first instance of the variable 'x', since it has the 'var' keyword.
In more complicated scripts, this could be useful. However, I tried some coding examples on my own, and it doesn't appear to make a difference either way for the script to run properly.
Hope that helps!
Dave StSomeWhere
19,870 PointsDave StSomeWhere
19,870 PointsAlso, the difference between let and var is Block Scope
Probably worth it to take the courses that show up from a
const
andlet
search. Defining variables with let and const workshop might be a good place to start.