Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial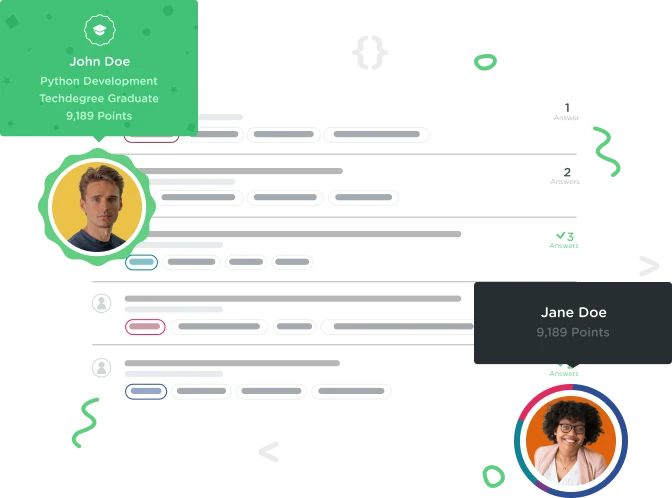

yoav green
8,611 Pointswhy do we need to "return item" in order to get { name: 'paper towels', price: 6.99 } eventually
const products = [
{ name: 'hard drive', price: 59.99 },
{ name: 'lighbulbs', price: 2.59 },
{ name: 'paper towels', price: 6.99 },
{ name: 'flatscreen monitor', price: 159.99 },
{ name: 'cable ties', price: 19.99 },
{ name: 'ballpoint pens', price: 4.49 }
];
const newArray = products
.filter(item => item.price < 10)
.reduce((highest, item) => {
if (highest.price > item.price) {
return highest
}
return item
},)
console.log(newArray);
1 Answer
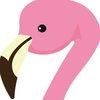
Dave StSomeWhere
19,870 PointsIf you review the MDN Doc you notice that it says:
accumulator The accumulator accumulates the callback's return values; it is the accumulated value previously returned in the last invocation of the callback, or initialValue, if supplied (see below).
So as the callback function loops through the array, when the next array item (the "item" in return item) is a higher value - return that value and reset the accumulator.
This should be clearer if you add some logging.
Like this:
const products = [
{name: 'hard drive', price: 59.99},
{name: 'lighbulbs', price: 2.59},
{name: 'paper towels', price: 6.99},
{name: 'flatscreen monitor', price: 159.99},
{name: 'cable ties', price: 19.99},
{name: 'ballpoint pens', price: 4.49}
];
const newArray = products
.filter(item => item.price < 10)
.reduce((highest, item) => {
console.log('Highest is --> ' + highest.price);
console.log('item is --> ' + item.price);
if (highest.price > item.price) {
console.log('about to return highest price --> ' + highest.price);
return highest
}
console.log('about to return item --> ' + item.price);
return item
},);
console.log(newArray);
// output
Highest is --> 2.59
item is --> 6.99
about to return item --> 6.99
Highest is --> 6.99
item is --> 4.49
about to return highest price --> 6.99
{ name: 'paper towels', price: 6.99 }
Does that clear things up?
yoav green
8,611 Pointsyoav green
8,611 Pointsyes, thanks a lot :)