Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial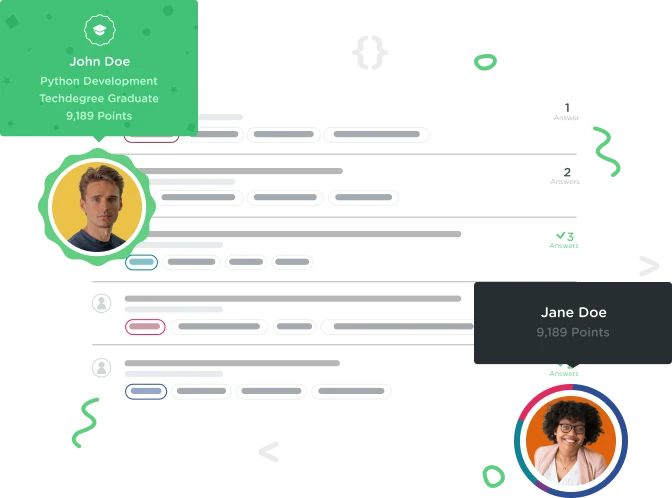
Kevin Frostad
3,483 PointsWhy do we need to use static for this task?
Since the variable is a public constant field it can be accessed from any of the class instances anyway. And since it's final, static wouldn't be necessary since we're not about to change its value, because we can't. What have I gotten wrong here?
2 Answers

James Simshaw
28,738 PointsHello,
What static does is allow you to use the variable without creating an instance of the object. For example, if I have a class:
public class MyClass {
public static final int CONSTANT_INTEGER = 42;
public final int mConstant_Final = 32
}
You can then access CONSTANT_INTEGER by doing
MyClass.CONSTANT_INTEGER
Yet, you cannot do the same with mConstant_Final, you must have an instance in order to access that value.
I hope this clears things up for you. If not, feel free to ask more questions and myself or someone else can hopefully clear things up more.
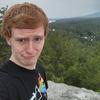
Brendon Butler
4,254 PointsSniped ;)
Kevin Frostad
3,483 PointsWouldn't doing this: MyClass myclass = new MyClass(); myclass.CONSTANT_INTEGER Be exactly the same as doing this: MyClass().CONSTANT_INTEGER;

James Simshaw
28,738 PointsBy doing MyClass.CONSTANT_INTEGER, do note there are no () after MyClass, you are not creating a new instance of MyClass and so not using up more memory.
Kevin Frostad
3,483 PointsSo whenever we want to use a method in another subclass we should also use static to save memory, which means every public method should also be static. Is there an exception to this, other than if you're aiming to use another object with the same name as the class?

Craig Dennis
Treehouse TeacherHi Tor!
Actually static and final should be separated in your brain. Sounds like you got final nailed down.
Static means whatever is being modified can be accessed at the blueprint, or class level. So you very well could keep a counter of how many times a class was instantiated by keeping a static field on the class. It should read "belongs to the class" in your brain versus belonging to the instance.
That help clear things up?
Kevin Frostad
3,483 PointsOk, so by using static on the instance (variable) CONSTANT_INTEGER you give full "ownership" of it to its class. So instead of asking both the class and the instance for it, you only ask the class. I just gotta say, Craig, your videos are awesome!
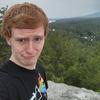
Brendon Butler
4,254 PointsLet's say you have a class named Chair. Inside that class you have a constant variable MAX_CAPACITY.
Without it being a static variable, in order to access it. You would have to create an instance of that class:
Chair chair = new Chair();
chair.MAX_CAPACITY;
Now, if you make that constant variable static you wont need to create an instance of the class to access the variable:
Chair.MAX_CAPACITY;
Just cleaner, doesn't waste system resources by creating a whole new object just to access a variable. There are some other benefits and drawbacks, but I want this answer to be as simple as possible. If you need more information you can read up on the Understanding Class Members documentation provided by Oracle.
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsHi Tor,
here is a nice link to this topic:
https://www.caveofprogramming.com/java/java-for-beginners-static-variables-what-are-they.html
Grigorij