Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial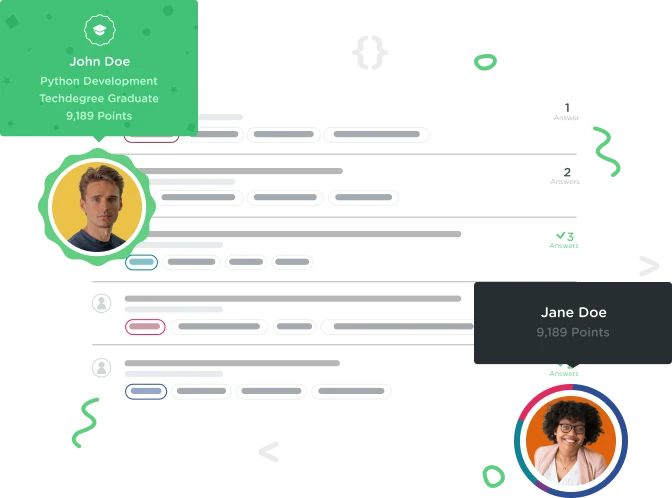
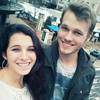
Alexander Mooney
7,321 PointsWhy do we use attr_writer instead of attr_accessor?
Wouldn't it be simpler to just use attr_accessor instead of writing out a reader for each attribute? Am I misunderstanding how accessor, reader, and writer work?
class Contact
attr_writer :first_name, :middle_name, :last_name
def first_name
@first_name
end
def middle_name
@middle_name
end
def last_name
@last_name
end
def full_name
full_name = first_name
if !@middle_name.nil?
full_name += " "
full_name += middle_name
end
full_name += ' '
full_name += last_name
full_name
end
end
2 Answers

Bill Rogers
12,944 PointsForgive me if I'm wrong, but I'm pretty sure you are correct in that, in this context, accessor would give you:
def first_name=(value)
@first_name = value
end
and
def first_name
@user
end
Whereas writer gives you the first method above, and then you write out the second by hand. Could be for practice?
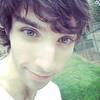
Alphonse Cuccurullo
2,513 PointsWhats with the += stuff in this syntax? Couldnt you just have did the + symbol? also why did you input end after the @middle name? Im having problems understanding the order of all this syntax someone help please?

Rodrigo Castro
15,652 PointsHi Alphonse,
Writing 'full_name += last_name' is the same as writing 'full_name = full_name + last_name', however the former is shorter. The 'end' after the @middle_name is used to close the method body which will return the @middle_name variable.
hope it helps.
Alexander Mooney
7,321 PointsAlexander Mooney
7,321 PointsI thought that may be it, but wanted to confirm rather than just assuming and being wrong. Thanks for the answer!
Tiago Ferrão
10,873 PointsTiago Ferrão
10,873 Pointsindeed! this is how I wrote the whole class in less than 10 lines and it seems to work with the same behaviour:
class User attr_accessor :first_name, :middle_name, :last_name
def full_name if middle_name.nil? full_name = first_name + " " + last_name else full_name = first_name + " " + middle_name + " " + last_name end end end