Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial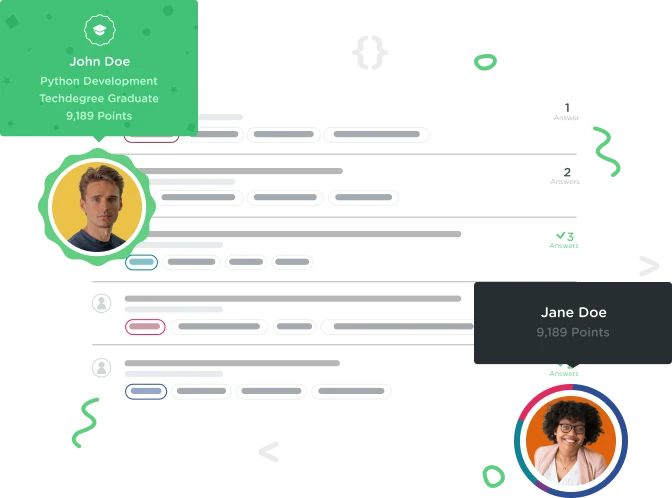

Faradja Nondo
1,485 PointsWhy do we use func createFriend(dict: [String: String]) -> Friend? instead of func createFriend(dict: String) ->?
On the following code:
struct Friend {
let name: String
let age: String
let address: String?
}
func createFriend(dict: [String: String]) -> Friend? {
if let name = dict["name"], let age = dict["age"] {
let adress = dict["address"]
return Friend(name: name, age: age, address: adress)
} else {
return nil
}
}
Why do we use
func createFriend(dict: [String: String]) -> Friend?{}
instead of
func createFriend(dict: String) ->?
or
func createFriend(name: String, age: String, address: String) -> Friend?{}
?
2 Answers
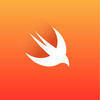
Steven Deutsch
21,046 PointsHey Faradja Nondo,
So your question is regarding the type of parameter we pass into the createFriend function. We are passing a dictionary in as the argument for this function, and then extracting the information from this dictionary for each key, inside of the functions body. The values for each key are then used to create an instance of friend.
If we used a parameter of type string, we would be lacking sufficient information to create our Friend instance:
/* It would be very difficult to create an instance of Friend with a single string
because we need to initialize 3 properties: name, address, and age
from this single string, because it is all the information the function has */
func createFriend(dict: String) -> Friend? {}
Passing the function 3 string parameters, provides sufficient information, and is perfectly valid!
func createFriend(name: String, age: String, address: String) -> Friend? { }
The reason why the instructor chose to use a dictionary as the parameter is because of the scope of this course. You are learning about the concept of optionals. Whenever you access a value in a dictionary by using a key, the value that is returned will be wrapped in an optional. This is because it is possible for you to look up a value using an invalid key, which would fail. You will be dealing with data in the form of dictionaries quite often, whether it be your own data model or data provided from an API, so this is an important concept to learn.
Good Luck!
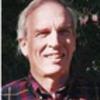
jcorum
71,830 PointsWe cannot use:
func createFriend(dict: String) -> Friend? { }
because a dictionary is not a String. We cannot use:
func createFriend(name: String, age: String, address: String) -> Friend? { }
because the function createFriend() needs to accept a dictionary as its parameter, not three Strings.
Just as an array of Strings has this type:
[String]
A dictionary whose keys and values are Strings has this type:
[String : String]
Note that the keys and values of a dictionary do not have to be of the same type. So you can have a dictionary whose keys are Strings, but whose values are Ints:
[String : Int]
Or the keys could be Ints and the values Strings:
[Int : String]
Using only one type to show the type of a dictionary would require keys and values to be of the same type.