Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial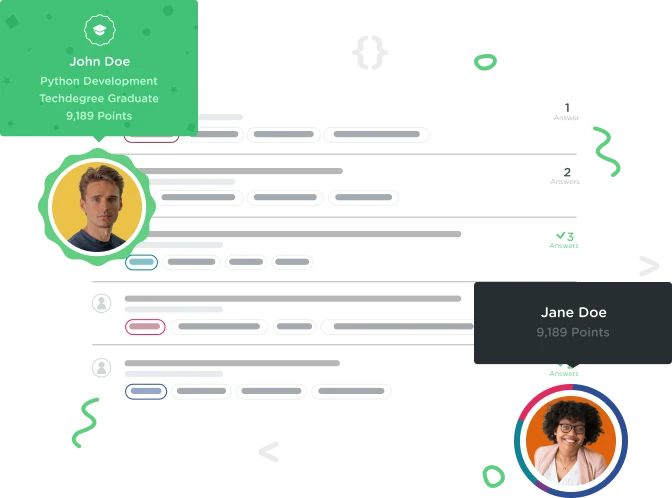

Kshatriiya .
1,464 PointsWhy do we use Object obj as an argument in compareTo(Object obj) if we're comparing two tweets?
public int compareTo(Object obj){}
This bit is really confusing me. Is the (Object) declaration inside compareTo(), a Superclass of the Tweet class?
This
Treet other = (Treet) obj;
is downcasting right? so (Object obj) must sit on top of the class hierarchy but you're just comparing two tweets which are clearly objects from Tweet class so why can't it be
compareTo(Tweet twt) {}?
If you look at this code it compares students and call the student object inside the method
public class Student implements Comparable {
private String studentname;
private int rollno;
private int studentage;
public Student(int rollno, String studentname, int studentage) {
this.rollno = rollno;
this.studentname = studentname;
this.studentage = studentage;
}
...
//getter and setter methods same as the above example
...
@Override
public int compareTo(Student comparestu) {
int compareage=((Student)comparestu).getStudentage();
/* For Ascending order*/
return this.studentage-compareage;
/* For Descending order do like this */
//return compareage-this.studentage;
}
How is this different to comparing tweets?
Also, is the compareTo() method called inside Arrays.sort()? If not where is it called?
Arrays.sort(tweet1.compareTo(tweet2)) //something like this maybe?
Thank you for your help.
1 Answer

Seth Kroger
56,413 PointsThere are essentially two forms of the Comparable interface, Comparable and the one using generics Comparable<Type>. In the video, Craig said he wasn't going to use generics because you haven't gotten to them yet in the course. So you are using the first form which has to take Object as a parameter to compareTo(). Since the interface has to work with any class, but doesn't have any knowledge of your class, it has to use the base Object class.
Your example Student class that passes a Student instead? It doesn't compile; I've tried. It will only compile right if you change the parameter type from Student to Object.
You can also use the generic form as well, and that way you would pass in a Treet. You just can't mix the two forms up.
public class Treet implements Comparable<Treet> {
//...
@Override
public int compareTo(Treet other) {
// ...
}
}
As to the second question: compareTo() is called deep in the inner workings of Arrays.sort(). As long as the objects in the array are from classes that implement Comparable, it will work fine.
Kshatriiya .
1,464 PointsKshatriiya .
1,464 PointsHi thank you for your help. If compareTo(Object obj) only accepts Object arguments, then shouildn't Array.sort() only accept object as well because presumably: