Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial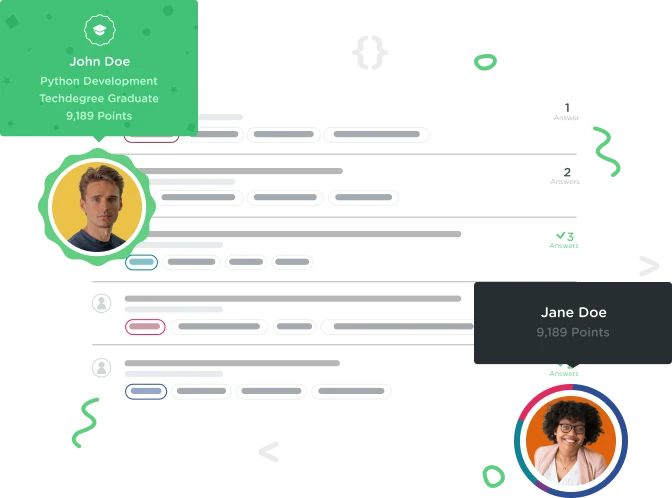

Yan Kozlovskiy
30,427 PointsWhy do we use prototypes in JavaScript?
I would like to know why we use prototypes. I understand that each time we create a new instance of a function, it'll create new space in memory. Are all elements attached to this prototype object? Why and when should we use something.prototype.somethingelse?
2 Answers
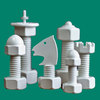
Steven Parker
231,275 PointsBasically, prototypes are a way of establishing properties and methods that will be inherited, or adding new properties and methods to an existing object or set of objects of a given type.
Now I realize that probably isn't much help in itself, but I found this article and discussion thread: JavaScript Prototype in Plain Language over at JavaScriptIsSexy.com that might be more enlightening.
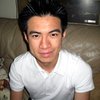
jason chan
31,009 PointsWe use prototypes for inheritance.
Since javascript isn't a formal object oriented language. That's how we do inheritance.
function Person(first, last) {
console.log(this);
this.firstname = first;
this.lastname = last;
console.log("This function is invoked.");
}
// inheriting from Person constructor to create a new method getFullName
Person.prototype.getFullName = function() {
return this.firstname + ' ' + this.lastname;
}
var john = new Person('john', 'doe');
console.log(john.getFullName());
var jane = new Person('jane', 'doe');
Person.prototype.getFormalFullName = function(){
return this.lastname + ', ' + this.firstname;
}
console.log(john.getFormalFullName());
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Inheritance_and_the_prototype_chain
// emca2015 you have to use babel to compile this to javascript. This more traditional way create classes and inheritance. This also is similar to typescript
"use strict";
// we create a class here a blue print using the keyword constructor and class
class Polygon {
constructor(height, width) {
this.height = height;
this.width = width;
}
}
// we extend or inherit from Polygon with new methods. Basically we just adding new functions to Polygon, but we creating a new object called Square.
class Square extends Polygon {
constructor(sideLength) {
super(sideLength, sideLength);
}
get area() {
return this.height * this.width;
}
set sideLength(newLength) {
this.height = newLength;
this.width = newLength;
}
}
var square = new Square(2);