Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial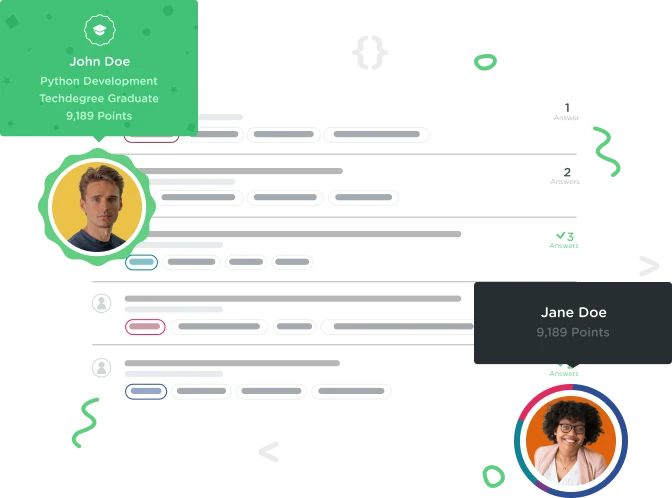
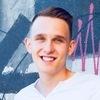
Gene Bogdanovich
14,618 PointsWhy do we use 'self' when referring to the property defined in the base class?
Given this chunk of code:
class Enemy {
var life: Int = 2
let position: Point
init(x: Int, y: Int) {
self.position = Point(x: x, y: y)
}
func decreaseLife(by factor: Int) {
life -= factor
}
}
class SuperEnemy: Enemy {
let isSuper: Bool = true
override init(x: Int, y: Int) {
super.init(x: x, y: y)
self.life = 50
}
}
In the init override in the SuperEnemy class, why do we use self to refer to the life property? It's defined in the base class and it was my understanding that we only use self to access properties in the class that we're currently in. I would appreciate any help!
2 Answers

Greg Kaleka
39,021 PointsHi Gene,
Oziel has it right, but let me see if I can simplify it a bit:
When a class inherits from another class, it automatically gets all of its properties and methods. By having : Enemy
in the class definition, SuperEnemy
gets the life
and position
properties as its own properties. It also gets the decreaseLife
method. This means that self.life
is perfectly fine because it is a property of the SuperEnemy
class.
Hope that helps!
Cheers
-Greg
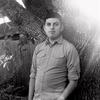
Oziel Perez
61,321 Pointsself does refer to the current class that you are in. But as far as I understand in swift, if the property isn't defined in the current class, but it is defined in the super class that it is derived from, then self will access that property. In contrast, if the property was written in the current class, then that property will override the super class property and self will have access to the property in the current class. This is helpful in case you want different classes to have different default values at initialization.
Disclaimer: I may have studied swift here, but I don't have much experience with the language, so my answer is based on experience with other OOP languages.
Gene Bogdanovich
14,618 PointsGene Bogdanovich
14,618 PointsThank you, Greg! That clears things up.
Greg Kaleka
39,021 PointsGreg Kaleka
39,021 PointsGlad to hear it - happy coding!