Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial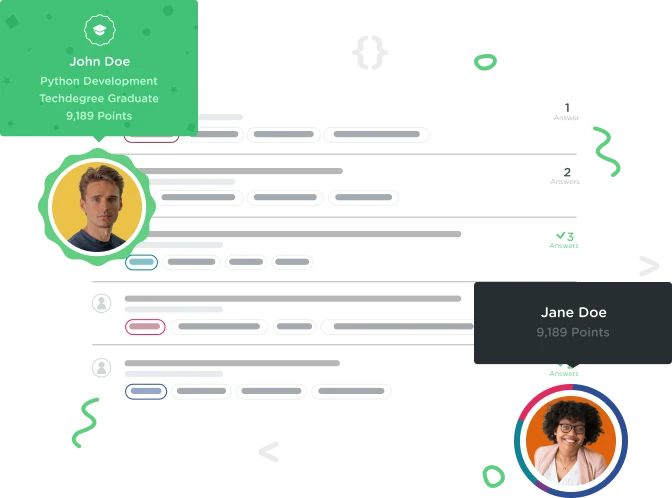

Alessandro Romani
19,723 PointsWhy do we use todo_item.push() and not @todo_item.push()?
I'm in doubt with instance variable @todo_item. I'm asking how we can push todo_items array in the add_item method if todo_items is local.

Alessandro Romani
19,723 PointsThis is the code: require "./todo_item"
class TodoList attr_reader :name, :todo_items
def initialize(name) @name = name @todo_items = [] end
def add_item(name) todo_items.push(TodoItem.new(name)) end
def remove_item(name) if index = find_index(name) todo_items.delete_at(index) return true else return false end end
def mark_complete(name) if index = find_index(name) todo_items[index].mark_complete! return true else return false end end
def find_index(name) index = 0 found = false todo_items.each do |todo_item| if todo_item.name == name found = true end if found break else index += 1 end end if found return index else return nil end end end
todo_list = TodoList.new("Groceries") todo_list.add_item("Milk") todo_list.add_item("Eggs") todo_list.add_item("Bread")
if todo_list.remove_item("Eggs") puts "Eggs were removed from the list." end
if todo_list.mark_complete("Milk") puts "Milk was marked as complete." end puts todo_list.inspect
3 Answers
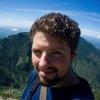
Garrett Carver
14,681 PointsHi Alessandro, When you add the line:
attr_reader :name, :todo_items
This allows you to use the variable without putting the @
symbol. Hope this helps. Let me know if you are still confused!
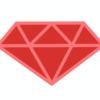
bashburn
7,611 Pointsattr_reader :todo_items creates the method:
def todo_items @todo_items end
Note that this method will return the array @todo_items.
The code in question todo_items.push(), calls the method todo_items which returns the array @todo_items, then the push method adds the new TodoItem object to the array.

Alessandro Romani
19,723 PointsBut with attr_reader can I set the variable todo_item pushing values? If is so what's the difference with attr_writer? Thank you for your help.
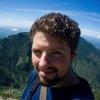
Garrett Carver
14,681 PointsI was not entirely sure about this either, but I found an article that explains it a little better. You can think of your objects as having various attributes. You want your methods to either 'get' or 'set' those attributes. So for example, if you want to set Object.color = "red"
then you use attr_writer
. On the other hand, if you only want to display the color when interacting with your object, then you would use attr_reader
, and return it by calling Object.color
. It's all about how you intend your objects to be interacted with when they are called.
Leo Brown
6,896 PointsLeo Brown
6,896 PointsThis was a while ago for me; can you please post a code sample?