Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial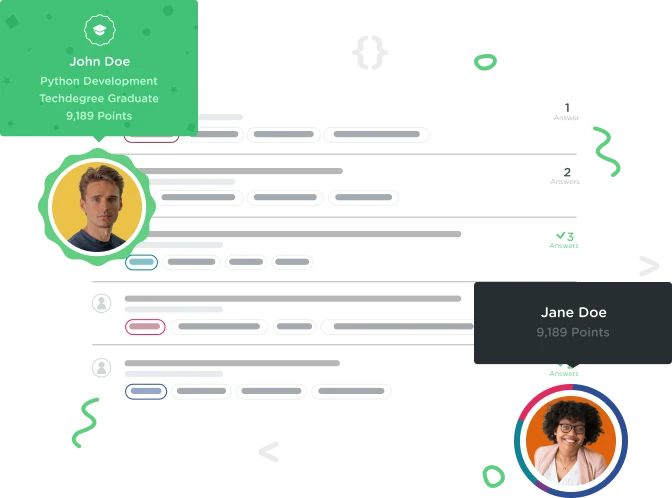
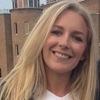
Sara Crwly
2,453 PointsWhy do you have to state the inputs after the method?
In this:
def get_name() print "Enter your name: " return gets.chomp end
def greet(name) print "Hi #{name}!" if (name == "Jason") puts "That's a great name!" end end
def get_number(number) print "What number would you like to test to see if it is divisible by 3? " return gets.chomp.to_i end
def divisible_by_3?(number) return (number % 3 == 0) end
name = get_name() greet(name) number = get_number()
if divisible_by_3?(number) puts "Your number is divisible by 3!" end
Why do you have to put?-
name = get_name() greet(name) number = get_number()
And why there and not at the end?
Thanks, S
1 Answer
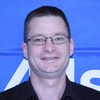
Seth Reece
32,867 PointsHi Sara,
The main reason for using methods, then calling them later is so they can be called over and over again. Then the method can be changed, and it's changed everywhere it is being used. get_number() is called where it is because it is assigning the return value to the variable number, then checking if that variable is divisible by three. A good example here would be if you also wanted to see if the number was divisible by 2. e.g.
def get_name()
print "Enter your name: "
return gets.chomp
end
def greet(name)
print "Hi #{name}!"
if (name == "Jason")
puts "That's a great name!"
end
end
def get_number()
puts "What number would you like to test to see if it is divisible by 2 and or 3? " # modified to check 2 and 3
return gets.chomp.to_i
end
def divisible_by_3?(number)
return (number % 3 == 0)
end
# new method to check if divisible by 2
def divisible_by_2?(number)
return (number % 2 == 0)
end
name = get_name()
greet(name)
number = get_number()
if divisible_by_3?(number)
puts "Your number is divisible by 3!"
else
# modified to give output regardless
puts "Your number is not divisible by 3!"
end
# new if statement to check for divisible by 2 using same number gotten from calling get_number()
if divisible_by_2?(number)
puts "Your number is divisible by 2!"
else
puts "your number is not divisible by 2!"
end
Sara Crwly
2,453 PointsSara Crwly
2,453 PointsThank you and the above clear and concise. I hadn't until now realised:
name = get_name() greet(name) number = get_number()
were used to call the methods over and over.