Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial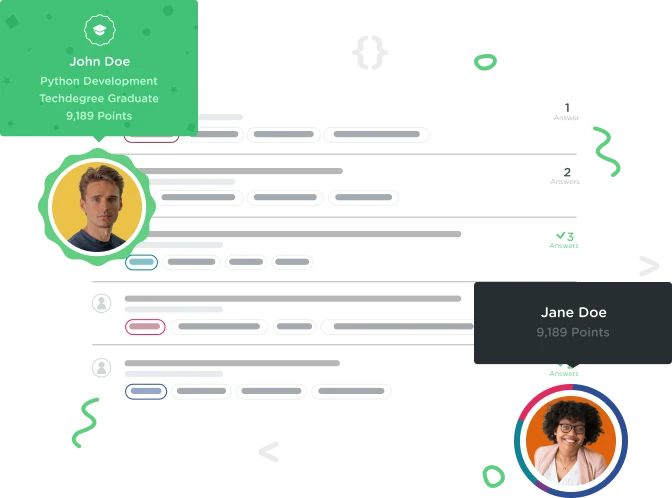
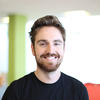
Kristian Woods
23,414 PointsWhy do you have to use a getter?
I understand why you would use a setter - to validate input before setting it, for example. However, why do you have to use a getter? cant you just set the property, then use dot notation to access the value?
let obj = {
set name(input) {
let name = input.split(' ');
this.firstName = name[0];
this.lastName = name[1];
},
firstName: 'Kristian',
lastName: 'Woods'
}
obj.name = 'John Smith';
console.log(obj.name);
why do you need the getter? I'm confused
Thanks
2 Answers
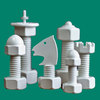
Steven Parker
231,269 PointsIn the video example, the "name" property is actually built from two separate stored fields, so it is necessary to compute and return the composite value.
Your code shown here will return "undefined" from the attempt to access obj.name, since there's no field by that name, and the getter has not yet been defined.

Luis Marsano
21,425 PointsI think this is one of those questions where the best answer is 'see for yourself'.
let test = { set name(input) { this.name = input } }
test.name = 'name'
Try it out and report back to us: you'll get an error. Why that particular error? Because you're using the setter inside itself, leading to unbounded recursion.
Even if you could make the setter assign a value without calling itself, e.g.,
test = { set name(input) {
Object.defineProperty(this, 'name', {configurable: true, enumerable: true, value: input})
console.log('still here')
} }
test.name = 'name'
test.name = 'name'
that overwrites the property and whatever accessor methods it had; notice the 2nd test.name = 'name'
no longer logs 'still here'.
A technical explanation is the language's specification of property: a property is exclusively either a data property (stores values) or an accessor property (getter and setter methods).
Never both.
This is typical of all languages offering similar object-oriented features.