Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial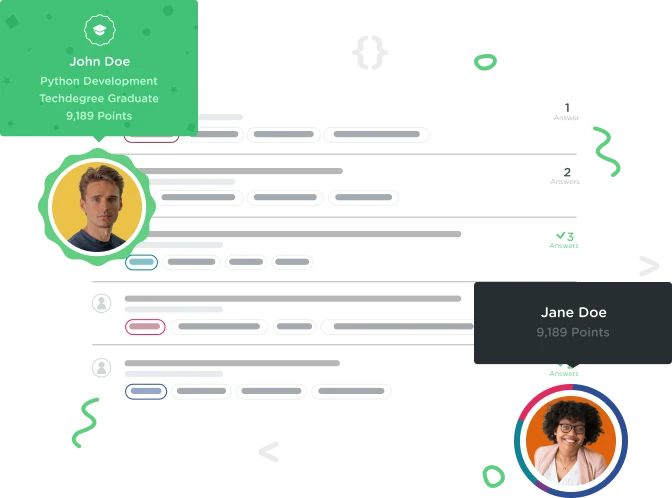

Barry Ort
12,373 Pointswhy do you need "+"
when assigning the valu of the rgb whith the value of the var "r" "g" and "b", why do you need to write rgb("+r+","+g+","+b+") and not just ("r","g","b")?
//Problem: No user interaction causes no change to application
//Solution: When user interacts cause changes appropriately
var color = $(".selected").css("background-color");
//When clicking on control list items
$(".controls li").click(function(){
//Deselect sibling elements
$(this).siblings().removeClass("selected");
//Select clicked element
$(this).addClass("selected");
//cache current color
color = $(this).css("background-color");
});
//When new color is pressed
$("#revealColorSelect").click(function(){
//Show color select or hide the color select
changeColor();
$("#colorSelect");
});
//update the new color span
function changeColor() {
var r = $("#red").val();
var g = $("#green").val();
var b = $("#blue").val();
$("#newColor").css("background-color", "rgb(" + r + "," + g +", " + b + ")");
}
//When color sliders change
$("input[type=range]").change(changeColor);
//When add color is pressed
//Append the color to the controls ul
//Select the new color
//On mouse events on the canvas
//Draw lines
<!DOCTYPE html>
<html>
<head>
<title>Simple Drawing Application</title>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8">
</head>
<body>
<canvas width="600" height="400"></canvas>
<div class="controls">
<ul>
<li class="red selected"></li>
<li class="blue"></li>
<li class="yellow"></li>
</ul>
<button id="revealColorSelect">New Color</button>
<div id="colorSelect">
<span id="newColor"></span>
<div class="sliders">
<p>
<label for="red">Red</label>
<input id="red" name="red" type="range" min=0 max=255 value=0>
</p>
<p>
<label for="green">Green</label>
<input id="green" name="green" type="range" min=0 max=255 value=0>
</p>
<p>
<label for="blue">Blue</label>
<input id="blue" name="blue" type="range" min=0 max=255 value=0>
</p>
</div>
<div>
<button id="addNewColor">Add Color</button>
</div>
</div>
</div>
<script src="//code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
1 Answer

Samuel Ferree
31,722 Pointsthe jQuery function .css() expects two parameters, both of which should be strings.
you need to convert your numerical r, g and b values, into a string of text, that meets the requirements of the rgb() css property.
The '+' operator, concatenates strings. consider the following code
let greeting = 'hello' + ' ' + 'world'; //=> 'hello world'
let r = 100;
let g = 200;
let b = 255;
let text = 'rgb(' + r + ',' + g + ',' + b + ');'; //=> 'rgb(100,200,255)'
Samuel Ferree
31,722 PointsSamuel Ferree
31,722 PointsIf you prefer something a bit more readable, ES5 gave us template literals. which can interpolate values by placing them inside of ${}
consider the following code:
Barry Ort
12,373 PointsBarry Ort
12,373 PointsOh I see. I didn'r realize that it took a string. thanx