Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial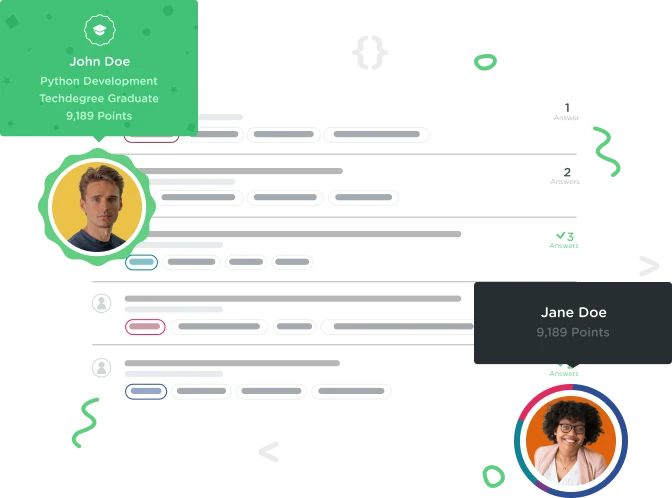
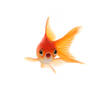
Robert Kwiat
5,349 PointsWhy do you need the variables a and b
He puts "int a" and "int b" in as the parameters of the function. Why can't you just put "int foo" and "int bar" into the parameters? Also, for the return statement, why can't you just put "foo" and "bar" in instead of "a" and "b". How do "a" and "b" even fit into the program. Thanks!
3 Answers

Aaron Arkie
5,345 PointsAs Adam Sackfield stated : he is using "a" and "b" as they are shorter than foo and bar. The entire code probably looks like this:
class example {
public static void main(String[] args){
//declare your function int funky_math(int a, int b); int main() { int foo = 24; int bar = 35; printf("funky math\n" +
funky_math(foo, bar)); return 0; }
int funky_math(int a, int b);
int main(){
int foo =24;
int bar =35;
system.out.println("funky math" + funky_math(foo,bar)); //this part is where the values of foo and bar are passed to a and b.
return 0;
}
}
}
what's going on here is a little bit of Algebra.
So what he's doing is called "passing" the values, it is annoying and confusing sometimes to keep track of the variables but its kind of like the transitive property of equality theorem: A=B & B=C SO A=C; or perhaps reflexive property of equality: A=A , B=B;
so in this case: a = foo b = bar
So rather than typing out foo and bar every time you right an equation we can substitute the variable names as A and B. But this also allows the int funky_math() method to remain unchanged manually whereas the variables of the main() method will mostly likely change throughout the program. It's just more efficient code to write it this way. I hope this helps, once you see this a couple of times it starts to click more just practice practice practice! let me know if i need to elaborate more (:

Aaron Arkie
5,345 PointsPlease post the code so i can have a look and we can work it out :)
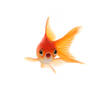
Robert Kwiat
5,349 Pointsinclude <stdio.h>
//declare your function int funky_math(int a, int b); int main() { int foo = 24; int bar = 35; printf("funky math %d\n", funky_math(foo, bar)); return 0; } int funky_math(int a, int b) {
return a + b + 343;
}
Adam Sackfield
Courses Plus Student 19,663 PointsAdam Sackfield
Courses Plus Student 19,663 PointsNot done anything on iOS. But I assume he is using "a" and "b" as they are shorter than foo and bar. A and B will be being used as variables.