Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial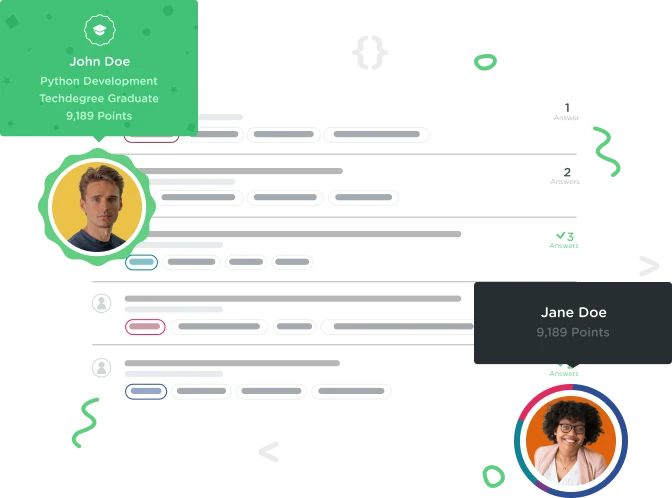
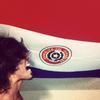
Sebastian Eguez
8,248 PointsWhy do you start with var correctGuess = false; ?
Why do you "assume the player didn't guess correctly" before starting the game? How can the player guess if the game hasn't started?
5 Answers
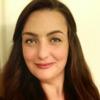
Jennifer Nordell
Treehouse TeacherI rewrote the code with comments to show you what happens when we don't initialize it to false. The end result is that it's impossible for the user to lose. Take a look:
// assume the player didn't guess correctly
// here's what would happen if we assumed they guessed correctly
var correctGuess = true;
// generate random number from 1 to 6
// for my purposes the computer has rolled a 5
var randomNumber = Math.floor(Math.random() * 6) + 1;
// for my purposes the user has guessed a 1
var guess = prompt('I am thinking of a number between 1 and 6. What is it?');
/* test to see if player is
1. correct
2. guessed too high
3. guessed too low
*/
if (parseInt(guess) === randomNumber ) { // this part doesn't execute because 1 is not equal to 5
correctGuess = true;
} else if (parseInt(guess) < randomNumber) { // this part would execute because 1 is less than 5
var guessMore = prompt("Try again. The number I am thinking of is more than " + guess + "."); //The user now guesses 2 which is still incorrect
if (parseInt(guessMore) === randomNumber) { // this doesn't execute because 2 is not equal to 5
correctGuess = true;
}
} else if (parseInt(guess) > randomNumber) { // this does not execute because 2 is not greater than 5 and the user will never be prompted again for a guess
var guessLess = prompt("Try again. The number I am thinking of is less than " + guess + ".");
if (parseInt(guessLess) === randomNumber) {
correctGuess = true;
}
}
// test if player is correct and output response
if (correctGuess) {
document.write ("<p>You guessed the number!</p>"); // This is what displays even though the user never guessed the correct number. By not initializing to false the user can never lose the game.
}
else {
document.write("<p>Sorry. The number was " + randomNumber + ".</p>");
}
edited for additional note
Your thinking is correct. It's not so much that it goes back to the initial value. The initial value was never changed at all if the user is wrong both times. But since it never changes when the user is wrong, we need to start it as being wrong. Otherwise no matter what they do they'll always be correct.
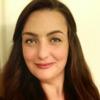
Jennifer Nordell
Treehouse TeacherIt's because the real start of the game happens when we prompt the user for their input. The correctGuess is only ever going to be true or false. This is declared and initialized before we prompt the user for their guess. It would be strange to automatically count their answer as correct before they've even given an answer. (Although, I could say that I've taken history exams where I wish that were the case.) So we assume they're incorrect. However, when they give us an answer then we look at it and if it is correct then we mark it correct.
It's a little like when you're taking a test. There's no teacher I've ever met that's automatically going to mark a blank question as correct just because they assume that if you haven't answered it must be correct.
Hope that makes sense!
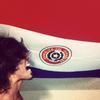
Sebastian Eguez
8,248 PointsHey Jennifer.
Is it because of the following in the end?
// test if player is correct and output response
if (correctGuess) {
document.write ("<p>You guessed the number!</p>");
}
else {
document.write ("<p>Sorry. The number was " + randomNumber + ".</p>");
}
Like this:
- It's first false (beginning).
- There's a bunch of scenarios where many things happen (middle)...
- It goes back to the initial false (beginning)(unless it was/is true) and you get the final "else" answer (end).
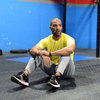
Juan Lugo
909 PointsThanks so much for clarifying this!
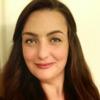
Jennifer Nordell
Treehouse TeacherYes, you could do that! But you'd have to rewrite the print statements or call a function to print the winning message instead. This makes our code less DRY. Here's a look at how it'd work without any correctGuess variable at all.
var randomNumber = Math.floor(Math.random() * 6) + 1;
var guess = prompt('I am thinking of a number between 1 and 6. What is it?');
if (parseInt(guess) === randomNumber) {
document.write('<p>You guessed the number!</p>');
} else if (parseInt(guess) < randomNumber) {
var guessMore = prompt('Try again. The number I am thinking of is more than ' + guess + '.');
if (parseInt(guessMore) === randomNumber) {
document.write('<p>You guessed the number!</p>');
}
} else if (parseInt(guess) > randomNumber) {
var guessLess = prompt('Try again. The number I am thinking of is less than ' + guess + '.');
if (parseInt(guessLess) === randomNumber) {
document.write('<p>You guessed the number!</p>');
}
else {
document.write('<p>Sorry. The number was ' + randomNumber + '.</p>');
}
}
edited for remove of extraneous comments in code
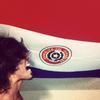
Sebastian Eguez
8,248 PointsGot it.
And there's a rule that says not to write a piece of code more than once.
You rock Jennifer!
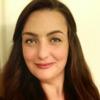
Jennifer Nordell
Treehouse TeacherIf we started with this:
var correctGuess;
... the underlying value, in fact, would be undefined
. This is a "falsey" value. Here is a list of JavaScript falsey values:
http://stackoverflow.com/questions/19839952/all-falsey-values-in-javascript. Now that being said, it wouldn't be explicitly equal to false. If we later did this comparison:
correctGuess === false;
The evaluation of that comparison would return false.
But the bottom line here is this: there is nothing besides the original declaration of the variable to ever change correctGuess to false. Which means correctGuess will only ever be true if we don't do that. This results in our user always being told they have the correct answer regardless of what they input.
At the bottom of this page at the bottom of "Add an Answer" is a link to a Markdown Cheatsheet that will teach you how to post your code like I post mine
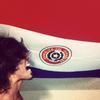
Sebastian Eguez
8,248 PointsI meant no var correctGuess; either. Nothing.
var randomNumber = Math.floor(Math.random() * 6) + 1;
That.

Liron Tal
4,825 PointsBecause as long as you didn't guess the correct number, the correctGuess is false.
Sebastian Eguez
8,248 PointsSebastian Eguez
8,248 PointsHow come your code appears in a black box and mine didn't?
What if we started with nothing? There wouldn't be an underlying answer sort of?