Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial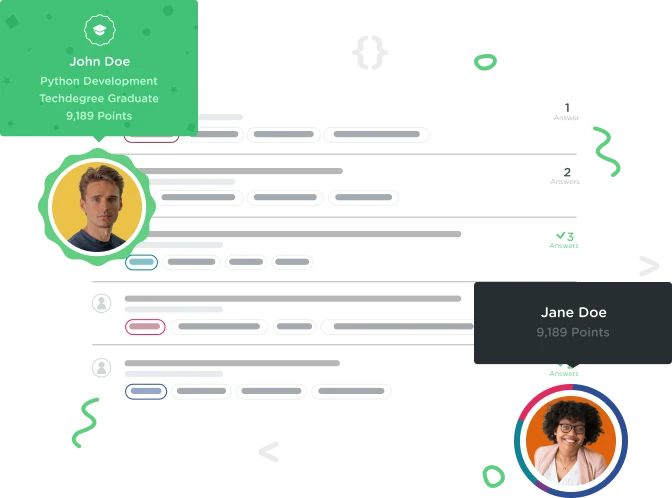
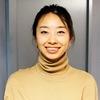
Flore W
4,744 PointsWhy does add(5,5) give 10, but if I define tuples = (5,5) and call the function add(tuples) it gives an error?
Following up on Kenneth example in the video, I have written:
def add(*nums):
total = 0
for num in nums:
total += num
return total
1st case, just as in the video returns 10
print(add(5,5))
2nd case as I am trying to define a separate variable and call the function on the variable, gives me "TypeError: unsupported operand type(s) for +=: 'int' and 'tuple'
tuples = (5,5)
print(add(tuples))
What's the difference between the two cases?
3 Answers
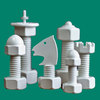
Steven Parker
231,269 PointsWhen you call "add(5,5)
", you are passing two number arguments to the function, which is what it is designed to handle.
But when you call "add(tuples)
", you are passing a single argument which is a tuple and not a number. This causes an error when the function internally tries to add the tuple to the numeric variable "total".
There is a built-in function named "sum" that will add together the values in an iterable, you could just call that instead: "sum(tuple)
".

Tilak Muruduru Divakar
6,459 PointsWhen you pass individual values like add(5,5), * in the function definition is packing these values into a touple, so that you are able to iterate over the touple and add each number to the total.
When you do add(tuples), * in the function definition is unpacking the touple you are passing into individual values.
You might wanna try add(*tuples). In this case "tuples" is unpacked and passed to the function as individual values which are packed again in the function definition.

Youssef Moustahib
7,779 PointsUse add(*tuples)
This will unpack the tuple which is like saying add(5,5)
Flore W
4,744 PointsFlore W
4,744 PointsThanks Steve, but I thought that the add function would take a tuple as argument, isn't it why there is the single * before nums? What does the *nums mean in that case? It only takes the values inside the tuple but not the tuple itself?
Steven Parker
231,269 PointsSteven Parker
231,269 PointsThe * ("gather" or "splat" operator) converts individual arguments into an interable that the loop can work with.
If you did not have the * then the function would work with the tuple, but not with the two (or more) numbers. And in that case it would be the same thing as "sum".
Flore W
4,744 PointsFlore W
4,744 PointsThanks, but in that case why don't we write the function as:
What is the advantage of using the * and the loop?
Steven Parker
231,269 PointsSteven Parker
231,269 PointsThis version would only work with two arguments. The version with the "splat" operator and the loop will work with any number of arguments.
Flore W
4,744 PointsFlore W
4,744 PointsGot it thanks!