Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial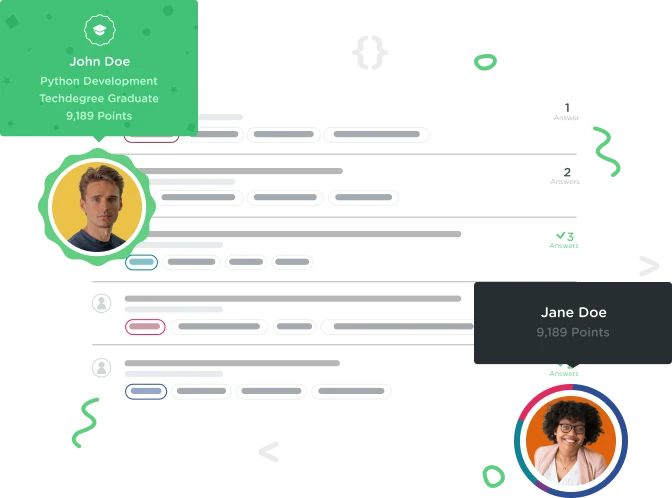

Joshua Gage
4,010 PointsWhy does adding value as an argument to the randomRGB function work?
Guill adds value as an argument to the randomRGB function, then replaces the randomValue function inside the parenthesis with said value. Why does this work? How does JS know to call randomValue() when Guill only added value as an argument to randomRGB?
How does JS know that randomValue() and value are the same thing?
3 Answers
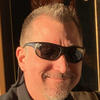
Peter Vann
36,427 PointsHi Joshua!
value is an arbitrary name for the function being passed in (as a parameter) here:
${randomRGB(randomValue)}
The give-away being this line of code:
const color = `rgb( ${value()}, ${value()}, ${value()} )`;
Which tells you that value is some kind of function because it's being used this way:
${value()}. // value is actually the function randomValue, because that is what is actually passed to randomRGB
In this line of code:
html += `div style="background-color: ${randomRGB(randomValue)}">${i}</div>`; // This is how JS makes the connection (how it "knows")
You could just as easily use this:
function randomRGB(callBackFunction) {
const color = `rgb( ${callBackFunction()}, ${callBackFunction()}, ${callBackFunction()} )`;
return color;
}
Or even this:
function randomRGB(x) {
const color = `rgb( ${x()}, ${x()}, ${x()} )`;
return color;
}
Or even this:
function randomRGB(anyName) {
const color = `rgb( ${anyName()}, ${anyName()}, ${anyName()} )`;
return color;
}
And it would work the same.
The name of the function variable as passed into the function randomRGB can be anything, as long as it is used consistently.
"value" just happens to be a good descriptive name semantically speaking.
Does that make sense?
I hope that helps.
Stay safe and happy coding!
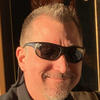
Peter Vann
36,427 PointsHi Joshua!
I thought I would simplify things even further, so. you can really see what's going on.
It possible that using a function as an argument/parameter is throwing you off, but it's perfectly common in JavaScript, especially for more advanced usages, such as callback functions and promises.
Consider this:
const square = function(num) {
return num * num;
}
console.log( square(2) );
Will log 4.
And this:
const square = function(num) {
return num * num;
}
const quad = function(func, num) {
return func(num) * func(num); // func is actually the square function and you are, in effect, squaring a square
}
console.log( quad(square, 2) ); // using square() inside quad
Will log 16.
In the first function (square), num = 2 when called in the log statement.
In the seceont function (quad), func = the square function, and num = 2 when called in that log statement.
But you could also do this:
const cube = function(num) {
return num * num * num;
}
const quad = function(func, num) { // This doesn't change
return func(num) * func(num); // func is actually the cube function in this case and you are, in effect, squaring a cube
}
console.log( quad(cube, 2) ); // using cube() inside quad (which is really 2**6, or 2 to th 6th power)
Will log 64.
Does that help you see it better?
I hope that helps.
Stay safe and happy coding!

Joshua Gage
4,010 PointsThank you!
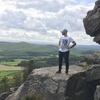
Jamie Moore
3,997 PointsI didn't ask the original question, but this helped me understand the concept a little better. Thanks for the detailed reply!
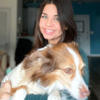
Robyn Patterson
Front End Web Development Techdegree Student 8,258 PointsMy code worked equally fine prior to passing the value to the argument, so why was this final step necessary? It feels like unneeded extra coding...
let html = '';
const randomValue = () => Math.floor(Math.random() * 256);
function randomRGB() {
const color = `rgb( ${randomValue()}, ${randomValue()}, ${randomValue()})`;
return color;
}
for (let i = 1; i <= 10; i++) {
html += `<div style="background-color: ${randomRGB()}">${i}</div>`;
}
document.querySelector('main').innerHTML = html;