Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial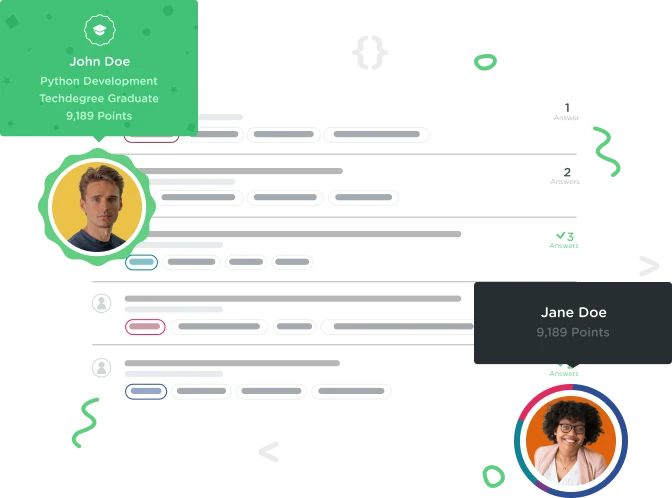

Janina Alvarez
8,083 PointsWhy does an arrow function not allow "this" access, but function() {} does?
In my solution to this part of the challenge, I provided:
const myString = { string: "Programming with Treehouse is fun!", countWords: () => { const words = this.string.split(' '); return words.length; }, }
this was giving me an error that this.string is undefined. When I changed it to the following, it passed:
const myString = { string: "Programming with Treehouse is fun!", countWords: function() { const words = this.string.split(' '); return words.length; }, }
Why is that the case?
const myString = {
string: "Programming with Treehouse is fun!",
countWords: () => {
const words = this.string.split(' ');
return words.length;
},
}
1 Answer
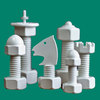
Steven Parker
231,275 PointsThe arrow function was designed to provide better support for object-oriented programming. With a conventional function, the scope is the function itself instead of the object. But within an arrow function, the this keyword refers to the object from which you define the function.
When used outside of an object (as in the example above), this refers to the global Window object.
Janina Alvarez
8,083 PointsJanina Alvarez
8,083 PointsThanks for your response, Steven Parker ! Can you add some clarification on your last statement, "When used outside of an object (as in the example above), this refers to the global Window object."
I thought because the function was defined within the object literal, it would be considered inside the object, not outside?
Steven Parker
231,275 PointsSteven Parker
231,275 PointsThe MDN page says that Arrow functions … inherit "this" from the parent scope at the time they are defined.
That same reference page warns that Arrow function expressions … cannot be used as methods … because they do not have their own "this". It goes on to illustrate the concept showing a code example very similar to your own.