Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial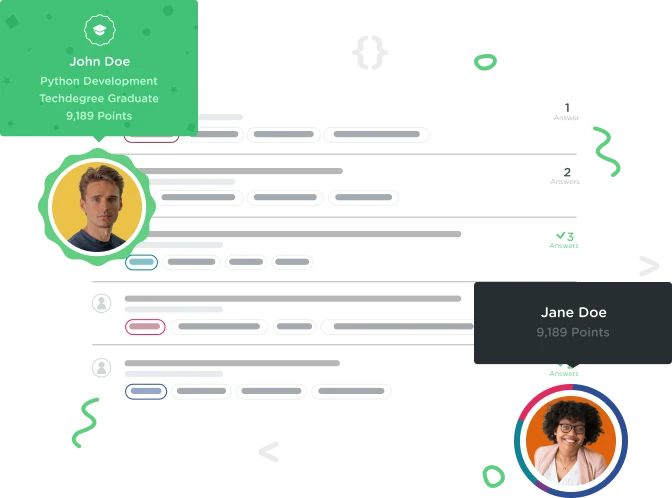

Hoessein Abd
Python Web Development Techdegree Graduate 19,107 PointsWhy does an attribute from a superclass change when an instance is made from a subclass?
So at around 4.16 in this video Kenneth makes an instance of the Thief class and gives it a name and sets sneaky to False. When an instance is made the init method will run from the Thief class and that sneaky will be set to False. Why does print(hoessein.hide(8)) result in False? The sneaky is True in the Sneaky class and also True in the Sneaky's init method.
from thieves import Thief
hoessein = Thief("Hoessein", sneaky=False)
print(hoessein.sneaky)
print(hoessein.agile)
print(hoessein.hide(8))
The output is:
False
True
False
I expected the sneaky only to be changed here when an instance is made from the thief's class:
import random
from attributes import Agile, Sneaky
from characters import Character
class Thief(Character, Agile, Sneaky):
sneaky = True
def __init__(self, name, sneaky=True, **kwargs):
super().__init__(name, **kwargs)
self.sneaky = sneaky
def pickpocket(self):
return self.sneaky and bool(random.randint(0, 1))
I didn't expect sneaky to change in the Sneaky class when I make an instance of the thief's class and set sneaky to false. Why does it do that?
import random
class Sneaky:
sneaky = True
def __init__(self, sneaky=True, *args, **kwargs):
super().__init__()
self.sneaky = sneaky
def hide(self, light_level):
return self.sneaky and light_level < 10
1 Answer

Ryan S
27,276 PointsHi Hoessein,
The sneaky attribute only defaults to True in the Sneaky __init__
method if a value is not provided. In the example given, you have overwritten the default by providing sneaky=False
, so self.sneaky
will be False as a result.
Hoessein Abd
Python Web Development Techdegree Graduate 19,107 PointsHoessein Abd
Python Web Development Techdegree Graduate 19,107 PointsThanks for your reply. I didn't supply enough information. I edited my original post.
Ryan S
27,276 PointsRyan S
27,276 PointsThe Thief class inherits from Sneaky, and it is in Sneaky's
__init__
method where it is possible to override the sneaky attribute value. So when an instance of Thief is created, thesneaky=False
value ultimately gets passed along to the Sneaky__init__
method, and will set the sneaky attribute for that instance of Thief.You aren't directly changing the sneaky value of the Sneaky class, you are setting the sneaky value in the Thief instance, which is inherited from the Sneaky class.
Hoessein Abd
Python Web Development Techdegree Graduate 19,107 PointsHoessein Abd
Python Web Development Techdegree Graduate 19,107 PointsAh, of course, i'm not changing the sneaky in the sneaky class, just the instance of the thieves class is sneaky. makes sense, thanks for the explanation.
Jay Reyes
Python Web Development Techdegree Student 15,937 PointsJay Reyes
Python Web Development Techdegree Student 15,937 PointsWhy do we need
sneaky = True
line under theclass Sneaky
?We know it's default to true when we instantiate the class.