Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial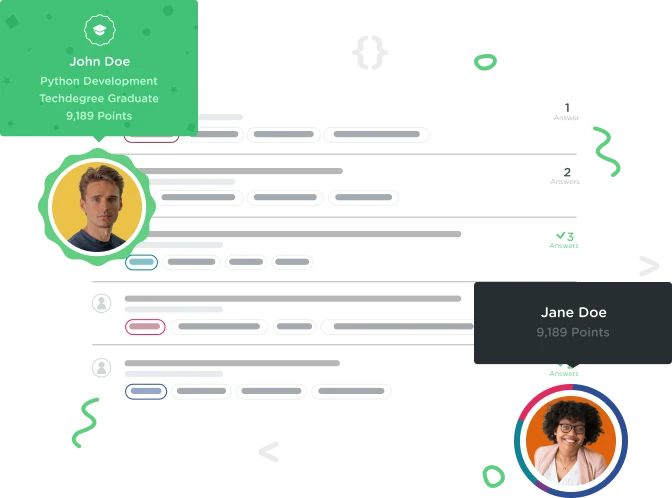
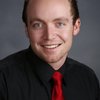
Justin Goldby
Full Stack JavaScript Techdegree Student 12,754 PointsWhy does Andrew chain the mouse event methods to the original $canvas.mousedown function?
I guess I am a bit confused as to why Andrew chains the mousemove mouseup and mouseleave methods to the canvas.mousedown function? How is it better than just making a new separate function with the same code in each of those functions, that is unchained to the previous method? Is this strictly for readability?
3 Answers
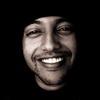
miikis
44,957 PointsHi Justin,
$canvas is a jQuery object; if you recall, you created it with:
// This wraps the DOM element "<canvas></canvas>" in a brand new object, with brand new properties and brand new methods that were created/attached by jQuery
// This brand new object is what we're calling a "jQuery object"
var $canvas = $('canvas');
So, as you're probably aware, some methods on a given jQuery object return actual...results, for lack of a better word. Like:
var width = $canvas.attr("width"); // In this case, this would return 800
Other times though, there are no results to be returned by a method. In such cases, a method could just return undefined/nothing but it's often desirable for a method to return the instance of it's parent object. Doing so allows the implementation of a Design Pattern known as "Chaining." Since chainable-methods are stupidly-simple to implement yet add astronomical points towards readability — and hence usability, (read: developer-sanity) — most/all? jQuery methods allow chaining when possible.
This is how simple this concept/pattern is:
function Person(firstname, lastname) {
this.firstName = firstname
this.lastName = lastname
}
Person.prototype.whoDis = function () {
// This method returns stuff, so this particular method is not chainable
return `I am ${this.firstName} ${this.lastName}!`
}
Person.prototype.sayMyNameSayMyName = function () {
// See, this method just logs stuff; it doesn't return anything
console.log(this.whoDis())
console.log(this.whoDis())
// So we can implement chaining!
return this // This returns a reference to the current instance of the Person constructor
}
var theDonald2 = new Person('John', 'Miller')
var theDonaldSpokesman = theDonald2
.sayMyNameSayMyName() // logs "I am John Miller!" twice; returns itself
.whoDis() // returns "I am John Miller!" — so theDonaldSpokesman variable would contain the value "I am John Miller!"
TL; DR
Yeah. For readability.

Ullas Savkoor
6,028 Points@Mikis Woodwinter : After going though your answer , certain things crossed my mind which I shall list below:
- You said that in method chaining the object returned by of one method is used by another method. This means that in chaining if I have Obj.method1().method2() then method2 is executed after method1 returns Obj.That is in chaining the order of execution is sequential ie it is as if the js file has 2 statements: obj.method1(); obj.method2(); 2.However if this chain of thought is applied to when eventhandlers are chained to an object(Jquery Object) as in like Obj.event1(function1).even2(function2), then shouldn't it mean event2 handler is called only after event1's handler return the object back?
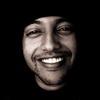
miikis
44,957 PointsOh I see what you're saying. And you're right to an extent: the code inside of Obj#event1 would be run before the code inside of Obj#event2. You're just forgetting that neither Obj#event1 nor Obj#event2 are calling the handlers directly. Rather, the purpose of both Obj#event1 and Obj#event2 is to attach the passed handlers — function1 and function2, respectively — as listeners to a particular DOM Event, like onclick. Then, when a user clicks on something, every listener attached to onclick is called. And, of course, a user clicking on something is asynchronous in nature. But you are right that the initial attaching of the event-listener is done synchronously/sequentially. Let me know if that made sense.

Ullas Savkoor
6,028 PointsThanks a lot :D, for getting my doubts even though my question was not very well formed. I had this doubt of event handling running in my head ever since I took up the Jquery Basics course with chaining of event handling. So to paraphrase you answer eventhandlers are getting binded to the element in a sequential order, thus repsonding to events would not be affected as they were binded in sequence and not called in a sequence.
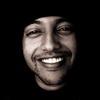
miikis
44,957 PointsYup, you got the gist of it. Glad I could help ;)