Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial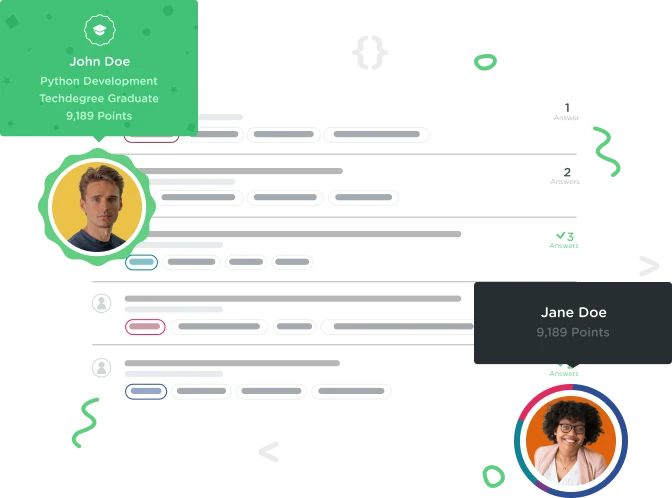

Yangzi He
2,125 PointsWhy does assigning the return value of the function to a constant created in the if condition solve the error message?
So given a correctly written isDivisible function, if we do:
<pre><code> if isDivisible(num1, num2) { \\ do something } else { \\ do other thing } </code></pre>
xCode gives an error that the optional type bool? cannot be used as a boolean. If we then instead do:
let result = isDivisible(num1, num2)
if result { \\ do something } else { \\ do other thing }
xCode gives the same error as above, saying that optional type bool? can't be used as a boolean. But how come the following code works without erroring?
if let result = isDivisible(num1, num2) { \\ do something } else { \\ do other thing }
Why does assigning the return value of the isDivisible function to a constant declare in the if condition pass the error check when the other two types don't?
1 Answer

Sérgio Leal
1,970 Pointsthe function isDivisible returns an optional isDivisible(num1, num2) -> Bool?
, so if you want to get it's value you have to unwrap it (using the !).
let result = isDivisible(num1, num2)
is an optional of type Bool? and not a Bool, to use it's value you need to unwrap it first.
// result is an optional of type Bool?
let result = isDivisible(num1, num2)
// result! is the value of the optional might be of type Bool or nil
if result! {
// Do something
} else {
// Do something else
}
the if let
it's an optional binding that makes it easier to test if an optional has a value.
if let result = isDivisible(num1, num2) {
// Do Something
} else {
// Do something else
}
In this conditional, the existence of a value returned by the function is tested first. If the returned value is nil, the else statement is executed. If is not nil, the optional returned by the function is unwrapped and assigned to the constant result.