Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial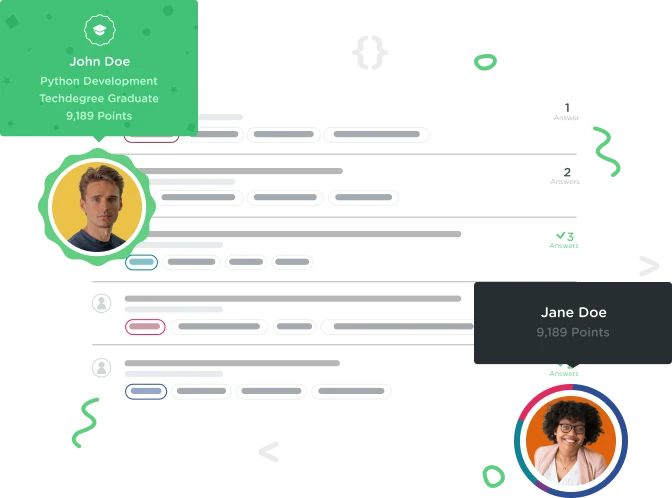

perix
795 PointsWhy does Craig copy the inventory without assigning a name to the copy?
At the end of the video, Craig uses this line of code:
for item in inventory.copy()
inventory.remove(item)
And when he accesses the inventory, there's nothing on the list.
>>> inventory
[]
Why did he made a copy if when accessing the Inventory(non copy) ,the for loop removed the items anyway ? And, if there's a way to access the copy that he made, how do you access it?
Also, why did he NOT assign a name to the copy so he could access it ?
1 Answer
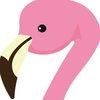
Dave StSomeWhere
19,870 PointsGood questions, sounds like you are paying attention, and this will be on a challenge
Why did he made a copy if when accessing the Inventory(non copy) ,the for loop removed the items anyway ? And, if there's a way to access the copy that he made, how do you access it?
Because removing items from a list your are iterating over will mess up the index (that's the concept being demonstrated)
Also, why did he NOT assign a name to the copy so he could access it ?
He doesn't need to access the copy. Only the for loop needs the copy, so a named isn't needed.
Here's some code to modify and test, that might help solidify the concepts:
inventory = ['item1', 'item2', 'item3']
inventory2 = ['2item1', '2item2', '2item3']
inventory3 = ['3item1', '3item2', '3item3']
print("\nLoop with copy list --> ", inventory, "\n")
for item in inventory.copy():
print('in loop item is ', item)
print(' inventory is ', inventory)
inventory.remove(item)
print("\ninventory after loop ", inventory)
print("\nLoop with copy list --> ", inventory2, "\n")
for item in inventory2:
print('in loop item is ', item)
print(' inventory2 is ', inventory2)
inventory2.remove(item)
print("\ninventory2 after loop", inventory2)
print("\nLoop with named copy list --> ", inventory3, "\n")
inventory3_copy = inventory3.copy()
for item in inventory3_copy:
print('in loop item is ', item)
print(' inventory3_copy is ', inventory3_copy)
print(' inventory3 is ', inventory3)
inventory3.remove(item)
print("\ninventory3 after loop ", inventory3)
print("\ninventory3_copy after loop ", inventory3_copy)
# output
Loop with copy list --> ['item1', 'item2', 'item3']
in loop item is item1
inventory is ['item1', 'item2', 'item3']
in loop item is item2
inventory is ['item2', 'item3']
in loop item is item3
inventory is ['item3']
inventory after loop []
Loop with copy list --> ['2item1', '2item2', '2item3']
in loop item is 2item1
inventory2 is ['2item1', '2item2', '2item3']
in loop item is 2item3
inventory2 is ['2item2', '2item3']
inventory2 after loop ['2item2']
Loop with named copy list --> ['3item1', '3item2', '3item3']
in loop item is 3item1
inventory3_copy is ['3item1', '3item2', '3item3']
inventory3 is ['3item1', '3item2', '3item3']
in loop item is 3item2
inventory3_copy is ['3item1', '3item2', '3item3']
inventory3 is ['3item2', '3item3']
in loop item is 3item3
inventory3_copy is ['3item1', '3item2', '3item3']
inventory3 is ['3item3']
inventory3 after loop []
inventory3_copy after loop ['3item1', '3item2', '3item3']
perix
795 Pointsperix
795 PointsOk, I get it. You're telling me you shouldn't remove an item from a list that is doing a for loop because it messes the indexes of the items, right?
About the copy, why does the remove function mess up the indexes of the items unless it is in a copy?