Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial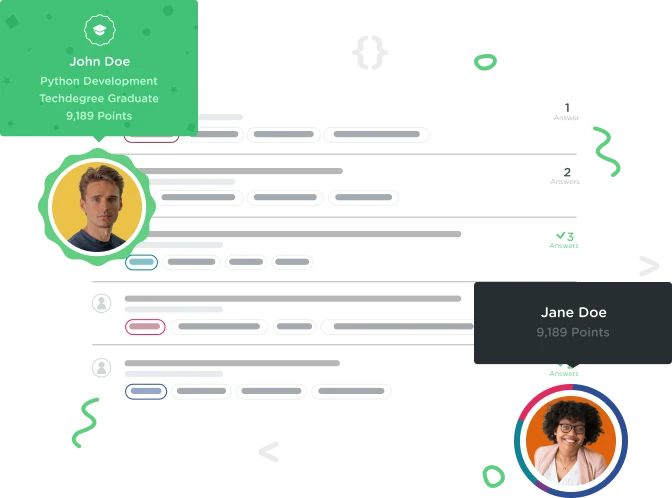
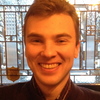
Andrew Smith
14,330 PointsWhy does "each" need to be redefined when the Enumerable module is included?
class Game
include Enumerable
attr_accessor :players
def each(&block)
players.each(&block)
end
def initialize
@players = []
end
...
def score
score = 0
players.each do |player|
score += player.score
end
score
end
end
In the method definition of each above, .each is being called. Since you can't define something by referring to itself, I'm guessing this is an override of the .each default definition (if not, somebody please tell me what the .each call is doing in its own definition). Why do we have to do this redefinition when the Enumerable module is included in a class? Why doesn't the default definition of .each pass in whatever calls it into a block?
1 Answer

Seth Kroger
56,415 PointsFrom what I see here, Game is not itself an Enumerable but does have a list (of players) that is. So in this case, instead of each() working on a list of Games it's redirecting to working on the list of players.
Andrew Smith
14,330 PointsAndrew Smith
14,330 PointsThanks for your reply. I vaguely understand that each is being redirected to work on the list of players instead of Games. What puzzles me in this method specifically:
is that the each method is being called within its own definition. How is this permissible? Is the each that is being called on the players array different than the each that is the name of the method being written here? Is the method written here being tacked on to or modifying a pre-existing each method in Ruby's collection of built-in methods?
Seth Kroger
56,415 PointsSeth Kroger
56,415 PointsYes, they are different methods from different classes with the same name. The one defined here is for the class Game, while players is of class Array/List (not sure which one) which has it's own definition of each.