Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial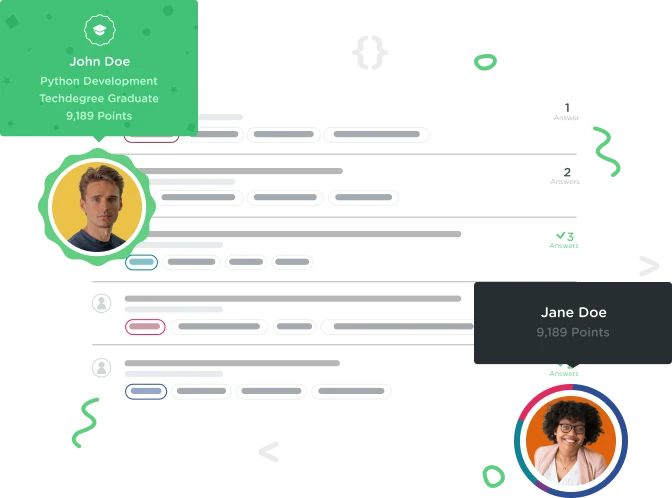

Arianna Saoirse
10,078 PointsWhy does every name return the 'not a student here' message?
All of the code here seems to work except the missing student message. That message prints whether the student is listed in the array or not.
var message = '';
var student;
var search;
var position = students.indexOf(search);
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport( student ) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt("Search student records: type a name (ex. = 'Dave') (or type 'quit' to end).");
if ( search === null || search.toLowerCase() === 'quit') {
break;
} else if (search === "" || search === " " ){
alert("Please enter a name");
} else if ( position < 0 ) {
alert("I'm sorry, '" + search + "' is not a student here. Please make sure the name is correct and try again.");
}
for (var i = 0; i < students.length; i += 1) {
student = students[i]
if ( student.name.toUpperCase() === search.toUpperCase() ) {
message = getStudentReport( student );
print (message);
}
}
}
2 Answers
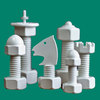
Steven Parker
231,268 PointsThere's no definition for "students". But even if it is defined elsewhere, when "position" is assigned on line 4, "search" was just created and is still undefined. So the result of "indexOf" will be -1.
Then later, since position is always less than 0, the message is always shown unless the input is empty or "quit". Even when a name is given, "position" is never updated.

Arianna Saoirse
10,078 PointsEnded up with this code which seems to work and didn't require much change:
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport( student ) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt("Search student records: type a name (ex. = 'Dave') (or type 'quit' to end).");
if ( search === null || search.toLowerCase() === 'quit') {
break;
} else if (search === "" || search === " " ){
alert("Please enter a name");
}
var position = -1;
for (var i = 0; i < students.length; i += 1) {
student = students[i]
if ( student.name.toUpperCase() === search.toUpperCase() ) {
message = getStudentReport( student );
print (message);
position = i;
break;
}
}
if ( position < 0 ) {
alert("I'm sorry, '" + search + "' is not a student here. Please make sure the name is correct and try again.");
}
}
Thanks for your help!
Arianna Saoirse
10,078 PointsArianna Saoirse
10,078 PointsThanks for your answer. Sorry, I should have included more information.
This is the code in it's entirety: https://w.trhou.se/ek2a0svmdv>
I forgot to mention that I also tried defining "position" in the while loop, with the same result:
(edit:) I have realized this is because it is an array of objects and the string returned does not match all of the properties in the object. So am I better changing my approach to this challenge, or should I create a for loop based on the search to find the object in the array which would return the correct position value?