Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial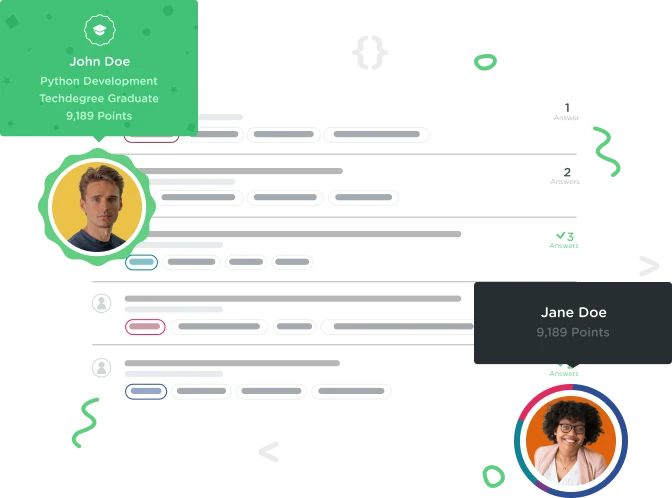
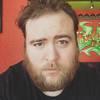
Michael Colmey
346 PointsWhy does "for" do what it does the way it does it?
I think I understand the basics of how for works, but I still don't understand why it does it the way it does. Take the overly simple statement below:
for name in names:
print(name)
If I remember correctly, this code should print each item defined in the names variable/list until their are no more and then end itself. The for function does this by putting each item in names into a new container/variable called name and then manipulating the new variable based on what you tell it to do on the next line; in this case simply print it.
I suppose part of what confuses me about this is why it has to have a new container/variable created to put an already existing list into. Why does "for names:" not work the same as "for name in names". It's manipulating the same exact data so why the extra step of putting it into a new container? Overall it feels like I get how to use for from a syntax perspective, but I don't really get the why behind how 'for' works like I do other statements such as if, not, elif, else, continue, break, etc.
2 Answers
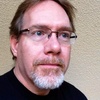
Chris Freeman
Treehouse Moderator 68,423 PointsLet's look at your questions.
> I suppose part of what confuses me about this is why it has to have a new container/variable created to put an already existing list into. Why does "for names:" not work the same as "for name in names". It's manipulating the same exact data so why the extra step of putting it into a new container?
The reason for the loop variable (new container) is to provide a reference to that object which can be used in other statements. If it were just "for names:
" then some default reference would be needed for the loop variable. In Perl they have $_
. In Python they have the saying "explicit is better than implicit." It also allows you to pick a readable, meaningful loop variable name like "for name in names:
", then use name
in the loop.
> Overall it feels like I get how to use for from a syntax perspective, but I don't really get the why behind how 'for' works like I do other statements such as if, not, elif, else, continue, break, etc.
Think of a for
loop as running the same block of code for each item within an iterable container such as a string, list, set, or dict. How can this be done?
First, create a pointer that will sequentially reference one item from the iterable at a time. Assign that pointer a name so it can be easily referenced by the block of code. When the code block is complete, advance the pointer and repeat until the iterable is exhausted.
There are two keywords that can redirect the flow of a for
loop.
-
continue
means stop the current iteration, advance the pointer and immediately start the next iteration. -
break
means stop all execution of thefor
loop and move to the next line of code following thefor
loop code block.
Post back if you need more details.
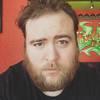
Michael Colmey
346 PointsThank you, its all making much more sense after reading your reply.