Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial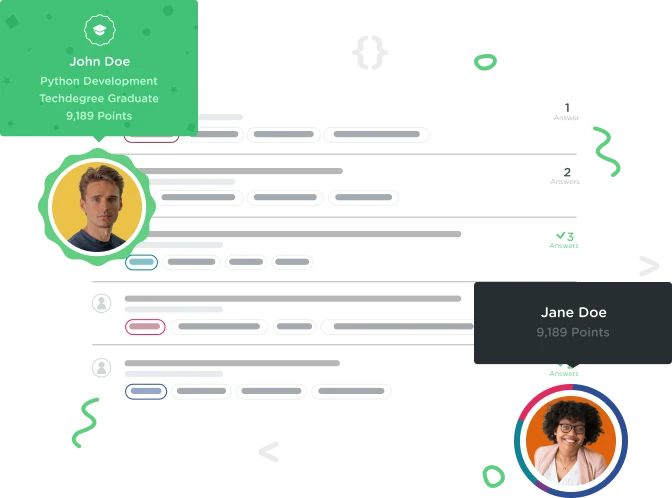

cchartm16
Courses Plus Student 2,464 Pointswhy does __init__ accept two arguments???
Why is it saying there are two arguments being passed to init???
class Student:
def __init__(self, *args):
self.name = "Your Name"
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
if grade > 50:
return self.praise()
return self.reassurance()
1 Answer

Chris Jones
Java Web Development Techdegree Graduate 23,933 PointsHey colinhartmann2,
self
is the Student object/instance that was created.
Perhaps this site will help some: http://www.diveintopython.net/object_oriented_framework/defining_classes.html
I found these two points helpful:
1) init is called immediately after an instance of the class is created. It would be tempting but incorrect to call this the constructor of the class. It's tempting, because it looks like a constructor (by convention, init is the first method defined for the class), acts like one (it's the first piece of code executed in a newly created instance of the class), and even sounds like one (βinitβ certainly suggests a constructor-ish nature). Incorrect, because the object has already been constructed by the time init is called, and you already have a valid reference to the new instance of the class. But init is the closest thing you're going to get to a constructor in Python, and it fills much the same role.
2) The first argument of every class method, including init, is always a reference to the current instance of the class. By convention, this argument is always named self. In the init method, self refers to the newly created object; in other class methods, it refers to the instance whose method was called. Although you need to specify self explicitly when defining the method, you do not specify it when calling the method; Python will add it for you automatically.
So, init is the first method that is called when creating an object. The self
parameter references the newly created object (a Student object in this case). *args
is a list of arguments you want to use in the init method. You also have **kwargs
, which is a collection of key-value pairs (ex: a dictionary) you can use in the init method.
Here's a site that talks about *args
and **kwargs
if you want to read more about them: https://www.saltycrane.com/blog/2008/01/how-to-use-args-and-kwargs-in-python/
I hope that helps!
Iain Simmons
Treehouse Moderator 32,305 PointsIain Simmons
Treehouse Moderator 32,305 PointsGreat answer!
Chris Jones
Java Web Development Techdegree Graduate 23,933 PointsChris Jones
Java Web Development Techdegree Graduate 23,933 PointsThanks, Iain!