Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial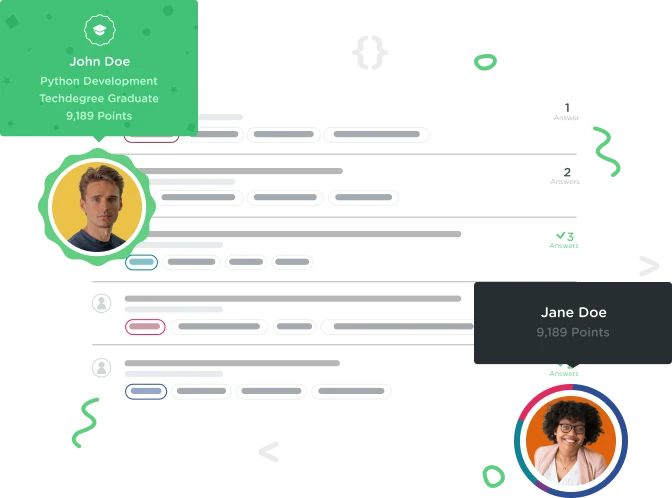

DaJuan Harris
Courses Plus Student 983 PointsWhy does insert(-1) insert the item at the beginning of the list.
I would think that the following code:
try:
#Position is now the absolute integer of itself
position = abs(int(position))
except ValueError:
position = None
if position is not None:
shopping_list.insert(position-1, item)
Would put the item at the end of the list if position was set to 0, because it would make position now be -1. It does what I want it do, but why is what I want to know.
Edit: I found out that if there is more than two items in the list, it doesn't put it at the beginning, but instead it will put it in front of the last item in the list. This just seems like a weird python oddity
1 Answer
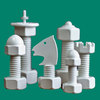
Steven Parker
230,274 PointsAn insert index of -1 will always put the new item just before the last item. That's because "-1" represents "the last item", and the insert function puts the new item before the one listed in the index.
DaJuan Harris
Courses Plus Student 983 PointsDaJuan Harris
Courses Plus Student 983 PointsYeah the user is choosing where to put the item in the list. It's being subtracted by 1, because of how indexing works. That's so if a user doesn't know how indexing works, they won't break the program. However, I do know how it works, so I was messing around with it. Below is the full code for the function
Steven Parker
230,274 PointsSteven Parker
230,274 PointsSo it seems a bit odd, but the function is actually performing consistently. The only way to cause "insert" to place an item at the end of a list is to use an index higher than the last item (such as the length of the list).