Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial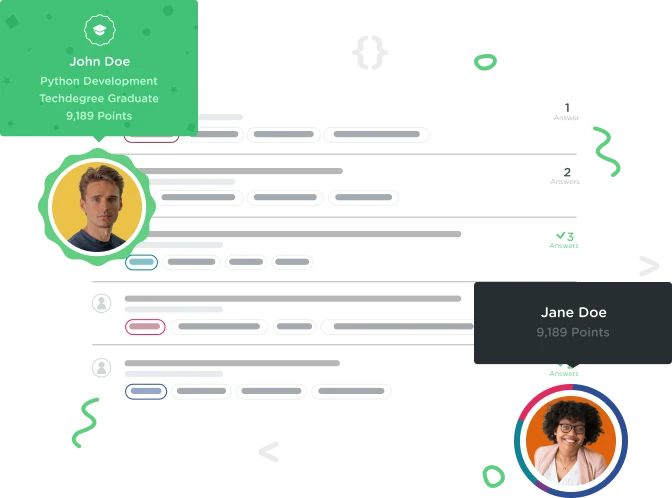

jackr
1,483 Pointswhy does int(0) == "random string" evaluate to true?
While playing around with conditionals, i found that int(0) returns true when using == comparison with ANY string. Only when I use the === operator does it properly evaluate false.
For example:
$string_one = 0; $string_two = "test string"; var_dump($string_one); // result is int(0) var_dump($string_two); // result is string(11)
if ($string_one == $string_two){
echo 'the values match';
} elseif ($string_one === ""){
echo '$string_one is empty';
} else {
echo 'the values do not match';
}
Running the above code will produce the result "the values match", which means that int(0) == string(11) returns true. This doesn't make sense to me - could someone please explain?
1 Answer

andren
28,558 PointsWhen you use == PHP will automatically convert the values you give it to the same type before it compares them. Since you are comparing an int and a string PHP automatically converts the string to an int. That's what allows comparisons like 42 == '42'
to work. If the string that is being converted does not actually contain any numbers PHP will just turn it into the number 0.
You can see this in effect if you manually cast the two variables as ints like this:
$string_one = 0;
$string_two = "what";
var_dump((int) $string_one);
var_dump((int) $string_two);
You will see that both of the var_dump
statement will output int(0)
.
This is not the only weird behavior you can encounter when using == to compare values, which is why === is usually the better operator to use if you want to be absolutely sure that the values are truly equal.