Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial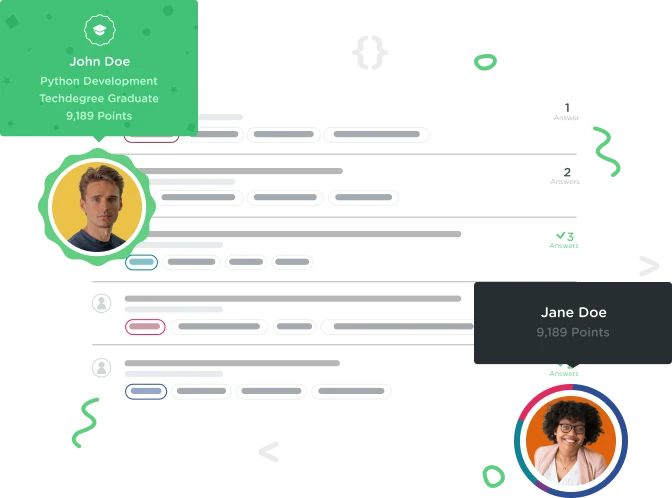
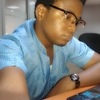
Nnamdi Orie
Courses Plus Student 5,284 Pointswhy does it add an empty string?
i noticed that when the input is empty and you click the 'add button' it adds a list of an empty string. how do i correct this.
1 Answer

andren
28,558 PointsIt adds an empty string because there is no code telling it not to. Computers do the exact thing your code tells it to and nothing beyond that. Since the code for adding an item simply instructs JavaScript to take the text value from the field and add it, that's what it does. Whether the text input is empty or not does not matter at all.
As for how to avoid that you just have to explicitly write code to check if the input is empty and stop the function if it is.
The simplest way of coding that would probably be something like this:
addItemButton.addEventListener('click', () => {
if (addItemInput.value.trim() === "") { // check if the (trimmed) input textbox is an empty string
return; // Return (stop the function) if that is the case
}
let ul = document.getElementsByTagName('ul')[0];
let li = document.createElement('li');
li.textContent = addItemInput.value;
ul.appendChild(li);
addItemInput.value = '';
});
There are two things in the above code you might not be familiar with. The first is trim()
, that is simply a method that removes white space (spaces, tabs, etc) from the start and end of a string. Without that you could enter a space into the text input box and that would be considered a non empty string and would therefore be added.
The second is that I use the return
keyword without specifying any value, this is because of the fact that I'm not using return in the way you are probably used to seeing it used. I'm not using it to pass some data from the function back to some other place, I'm simply making use of the fact that when a function returns it stops running, which means that all of the code below the return statement is not executed.
Nnamdi Orie
Courses Plus Student 5,284 PointsNnamdi Orie
Courses Plus Student 5,284 Pointswow!! what a relief. How did you become so good? i wanna be really good.
ywang04
6,762 Pointsywang04
6,762 PointsThanks a lot. :)