Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial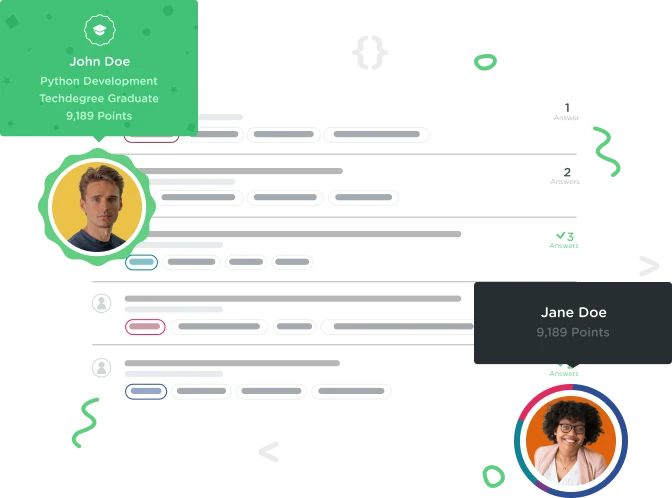

John Jones
Courses Plus Student 319 PointsWhy does it display the error dialog when I upload it to my tablet?
When I upload it to my tablet sometimes it will display the title with author and all the information but when I load it sometimes it doesn't display anything. To fix this I had to rotate it back and fourth from vertical to horizontal 10+ times for it to get the data. Is there something wrong with the code?
My internet is not down or anything but 80% of the time it will show the error dialog while the other 20% it will show the actual data from the treehouse blog. PLEASE HELP I HAVE BEEN ASKING THIS MULTIPLE TIMES HOPING TO GET THIS FIXED WITH NO ANSWERS ON ANYONE OF THEM.
P.S I also want to know if this problem persists on any other project that connects to the internet such as the next project "Build a self destructing app"
Here is my Code:
package com.example.blogreadingrepeat;
import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import java.io.Reader; import java.net.HttpURLConnection; import java.net.MalformedURLException; import java.net.URL; import java.util.ArrayList; import java.util.HashMap;
import org.json.JSONArray; import org.json.JSONException; import org.json.JSONObject;
import android.app.AlertDialog; import android.app.ListActivity; import android.net.ConnectivityManager; import android.net.NetworkInfo; import android.os.AsyncTask; import android.os.Bundle; import android.text.Html; import android.util.Log; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.ArrayAdapter; import android.widget.ProgressBar; import android.widget.SimpleAdapter; import android.widget.TextView; import android.widget.Toast;
public class MainListActivity extends ListActivity {
public static final int NUMBER_OF_POSTS = 20;
public static final String TAG = MainListActivity.class.getSimpleName();
protected JSONObject mblogData;
protected ProgressBar mProgressBar;
private final String KEY_TITLE = "title";
private final String KEY_AUTHOR = "author";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main_list);
mProgressBar = (ProgressBar) findViewById(R.id.progressBar1);
if(isNetworkAvailable()){
mProgressBar.setVisibility(View.VISIBLE);
GetBlogPostsTask getBlogPostsTask = new GetBlogPostsTask();
getBlogPostsTask.execute();
}
else{
Toast.makeText(this, "Network is down. ", Toast.LENGTH_LONG).show();
}
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager) getSystemService(CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;{
if(networkInfo != null && networkInfo.isConnected()){
isAvailable = true;
}
return isAvailable;
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main_list, menu);
return true;
}
public void handleBlogResponse() {
mProgressBar.setVisibility(View.INVISIBLE);
if(mblogData == null){
updateDisplayForError();
}
else{
try {
JSONArray jsonPosts = mblogData.getJSONArray("posts");
ArrayList<HashMap<String, String>> blogPosts = new ArrayList<HashMap<String, String>>();
for(int i = 0; i < jsonPosts.length(); i++){
JSONObject post = jsonPosts.getJSONObject(i);
String title = post.getString(KEY_TITLE);
title = Html.fromHtml(title).toString();
String author = post.getString(KEY_AUTHOR);
author = Html.fromHtml(author).toString();
HashMap<String, String> blogPost = new HashMap<String, String>();
blogPost.put(KEY_TITLE, title);
blogPost.put(KEY_AUTHOR, author);
blogPosts.add(blogPost);
}
String[]keys = {KEY_TITLE , KEY_AUTHOR};
int[] ids = {android.R.id.text1, android.R.id.text2};
SimpleAdapter adapter = new SimpleAdapter(this, blogPosts, android.R.layout.simple_list_item_2, keys, ids);
setListAdapter(adapter);
} catch (JSONException e) {
Log.e(TAG, "Json Exception Found: " , e);
}
}
}
private void updateDisplayForError() {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle(getString(R.string.title));
builder.setMessage(getString(R.string.Error_Message));
builder.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
TextView emptyTextView = (TextView) getListView().getEmptyView();
emptyTextView.setText(getString(R.string.No_items));
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
private class GetBlogPostsTask extends AsyncTask<Object, Void, JSONObject>{
@Override
protected JSONObject doInBackground(Object... params) {
int responseCode = -1;
JSONObject jsonResponse = null;
try {
URL blogFeedUrl = new URL("http://blog.teamtreehouse.com/api/get_recent_summary/?count=" + NUMBER_OF_POSTS);
HttpURLConnection connection = (HttpURLConnection) blogFeedUrl.openConnection();
connection.connect();
responseCode = connection.getResponseCode();
if(responseCode == HttpURLConnection.HTTP_OK){
InputStream inputStream = connection.getInputStream();
Reader reader = new InputStreamReader(inputStream);
int content = connection.getContentLength();
char[] charArray = new char[content];
reader.read(charArray);
String responseData = new String(charArray);
jsonResponse = new JSONObject(responseData);
}
} catch (MalformedURLException e) {
Log.e(TAG, "Malformed URL Exception Found: " ,e );
}
catch(IOException e){
Log.e(TAG, "IOExecption Found: " , e);
}
catch(Exception e){
Log.e(TAG, "Exception Found: " , e );
}
return jsonResponse;
}
@Override
protected void onPostExecute (JSONObject result){
mblogData = result;
handleBlogResponse();
}
}
}