Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial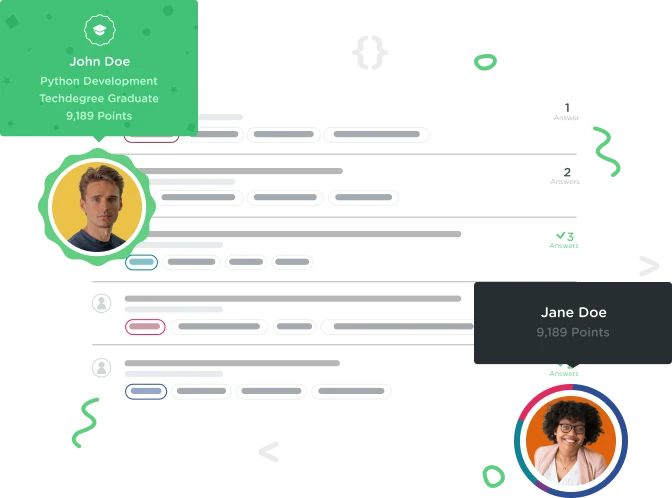

Jay Harper-Harrison
1,044 PointsWhy does it do this?
state_names = ["Alabama", "California", "Oklahoma","Florida"]
vowels = list('aeiou')
output = []
for state in state_names:
state_list = list(state.lower())
for vowel in vowels:
while True:
try:
state_list.remove(vowel)
except:
break
output.append(''.join(state_list).capitalize())
print(output)
My code prints this out: ['Lbm', 'Lbm', 'Lbm', 'Lbm', 'Lbm', 'Cliforni', 'Cliforni', 'Clforn', 'Clfrn', 'Clfrn', 'Oklhom', 'Oklhom', 'Oklhom', 'Kl hm', 'Klhm', 'Florid', 'Florid', 'Flord', 'Flrd', 'Flrd'] can someone help me?
2 Answers
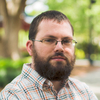
Kenneth Love
Treehouse Guest TeacherYour output.append
is at the same indentation as your while
, so you're appending for each vowel. Since there are 5 vowels, you get 5 appends. Put the output.append
at the same level as the for
and you shouldn't have that problem.

Andrew Molloy
37,259 PointsYou're looping through your append too, remove an indent for your output.append line to take it out of the while loop
Jay Harper-Harrison
1,044 PointsJay Harper-Harrison
1,044 PointsCould you explain why it appends for each vowel please?
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherJay Harper-Harrison Sure. Python uses indentation to see what code belongs inside what block instead of braces like many other languages. Let's rewrite part of yours as some pseudo-code that uses braces to show blocks.
As you see in the above example, your
output.append()
belongs to thefor
loop that's looping over the vowels. So the loop goes through with "a", removing it from the word, if possible, then appending the current state of the word to theoutput
list. Then it goes through the loop with "e", removing it if possible and appending. Then with "i". Then with "o", and so on.If you unindent, or dedent, your
output.append()
line by one tab/set of spaces, it'll be outside of thefor
loop and will only append once all of the vowels have been processed.