Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial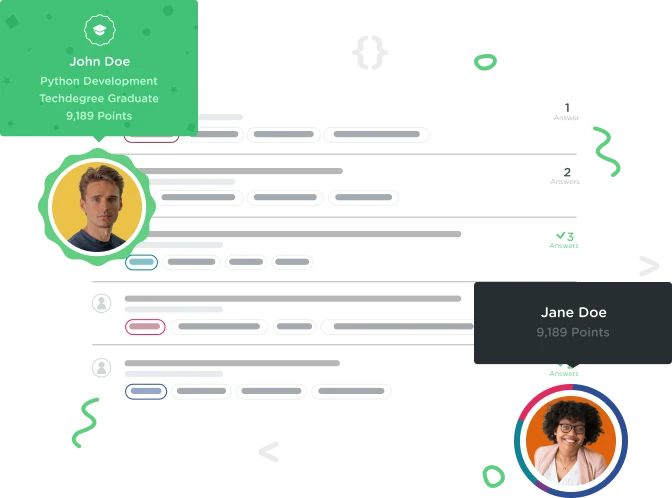

Casey Beaver
5,619 PointsWhy does it matter that we initially set our answer variables to false?
I was able to get this to work by setting the answers to false, but I was also able to get it to work without setting any correct answer variables.
Is initially setting each correct answer to false good practice for a reason? My initial solution with the variables set to false is commented out for comparison.
Can someone please shed some light on what I could encounter that would be problematic by not creating the correct answer 'ca' false variables?
I appreciate the help.
const a1 = 'CASEY'; const a2 = '32'; const a3 = 'MALE'; const a4 = 'BLACK'; const a5 = 'BASKETBALL';
//let [ca1, ca2, ca3, ca4, ca5] = [0];
let rank = '0';
let q1 = prompt("What's your name?"); let q2 = prompt("What's your age?"); let q3 = prompt("What's your gender?"); let q4 = prompt("What's your favorite color?"); let q5 = prompt("What's your favorite sport?");
if (q1.toUpperCase() === a1) { // ca1 = true; rank++; }
if (q2.toUpperCase() === a2) { // ca2 = true; rank++; }
if (q3.toUpperCase() === a3) { // ca3 = true; rank++; }
if (q4.toUpperCase() === a4) { // ca4 = true; rank++; }
if (q5.toUpperCase() === a5) { // ca5 = true; rank++; }
//if(ca1){rank++}; //if(ca2){rank++}; //if(ca3){rank++}; //if(ca4){rank++}; //if(ca5){rank++};
console.log(rank);
if (rank > 4){
document.querySelector('main').innerHTML = <h2>Congrats! you received gold for getting all ${rank} answers correct!</h2>
;
}
else if (rank >= 3 && rank < 5){
document.querySelector('main').innerHTML = <h2>Nice! You received silver for getting ${rank} answers correct!</h2>
;
}
else if (rank <= 2 && rank > 0){
document.querySelector('main').innerHTML = <h2>Decent. You received bronze for getting ${rank} answers correct.</h2>
;
}
else {
document.querySelector('main').innerHTML = <h2>Ouch. You received no crown for getting ${rank} answers correct.</h2>
;}
2 Answers
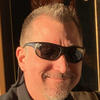
Peter Vann
36,425 PointsHi Casey!
I hope this answers your question adequately:
Have you ever heard of the concepts of truthy and falsy?
Basically, any condition that will NOT evaluate to true is falsy and any condition that will NOT evaluate to false is truthy.
So empty variables will, in effect, be falsy, because they will NOT evaluate to true.
More info:
https://www.sitepoint.com/javascript-truthy-falsy/
I hope that helps.
Stay safe and happy coding!
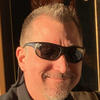
Peter Vann
36,425 PointsHi Casey!
Thanks for the kudos - it's my pleasure. It feels nice to be able to contribute!
It doesn't apply to your current question, but here is something to think about (which can relate to the whole truthy/falsy issue).
Whenever possible, name your variables (especially boolean-value variables) very descriptive and it will really make your code readable.
For example, instead of this:
if (result == True) {
// code
} else {
// alt code
}
You could do this:
if (user_answered_everything_correct) {
// code if user did
} else {
// code if user didn't
}
If user_answered_everything_correct happens to be true, it will execute, if it's false, null, or an empty string (any falsy value), it will not.
And by naming it user_answered_everything_correct, (user_answered_everything_correct == True) is kinda redundant and ever so slightly harder to read and understand.
Does that make sense?
I hope that helps.
Stay safe and happy coding!

Casey Beaver
5,619 PointsHi Peter,
That does make sense. Especially if I'm writing more complex or lengthy code that I have to step away from for awhile, or handing it off to other members of my team. I know I'd appreciate accurate variables if I was looking at someone else's work for the first time.
I always appreciate good advice and direction.
Thank you very much!
Casey Beaver
5,619 PointsCasey Beaver
5,619 PointsHi Peter,
Thank you so much for sending that my way. It helped clear up the confusion I had and solidify my learning foundation a bit more for moving forward.
Much appreciated. Cheers!