Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial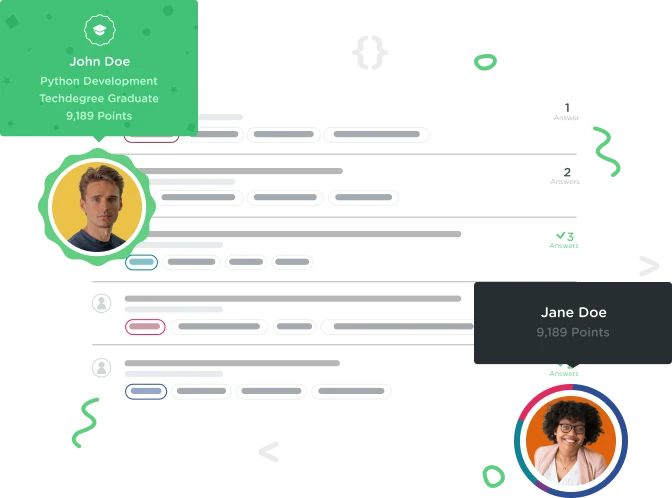
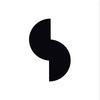
svenvanzijl
573 PointsWhy does it print A, D, F?
I don't understand why it prints A, D, F?
print("A")
try:
result = "test" + 5
print("B")
except ValueError:
print("C")
except TypeError:
print("D")
else:
print("E")
print("F")
2 Answers
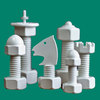
Steven Parker
229,732 PointsSince you know the answer, you should have seen this explanation when you selected it in the quiz: "Adding a string and an int raises a TypeError. Since an exception occurred the else block doesn't run."
If we turn that explanation into line-by-line comments:
print("A") # this prints the "A"
try:
result = "test" + 5 # adding a string and an int raises a TypeError
print("B") # so this line is skipped over
except ValueError: # this line is also skipped over
print("C") # this line is also skipped over
except TypeError: # the code resumes running here
print("D") # this prints the "D"
else: # since an exception occurred, the else block doesn't run
print("E") # so this line is skipped over
print("F") # finally, this prints the "F"
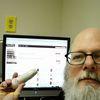
Rick Gleitz
47,197 PointsHi Svenvanzijl,
A and F are going to be printed in any case because they are outside the try/except/else portion of the code. The other choices are within the try/except/else part, so the only thing to figure out is which one. If the value of the variable result was valid, it would print B also (it is not valid). ValueError as I understand it is mostly for catching things that don't normally make mathematical sense, like taking the square root of a negative number, which is not what we have here, so it doesn't print C. It does print D because result tries to add two things that are mismatched types (an integer and a string). Lastly, it doesn't print E because it did catch an error. So it prints A, D, F.
Hope this helps.