Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial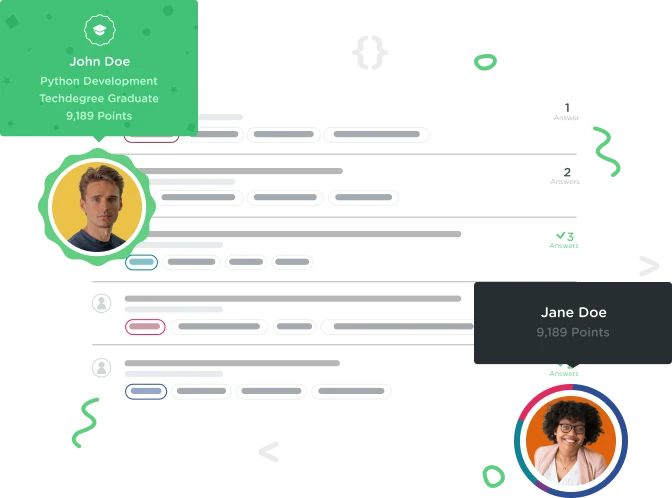

tytyty
4,974 PointsWhy does it proceed if the condition is true?
let cards = 1...13
for card in cards {
if card == 11 {
println("Jack")
} else if card == 12 {
println("Queen")
} else if card == 13 {
println("King")
} else {
println(card)
}
}
I'm confused as to why the output keeps going. I thought it's supposed to stop at 11/Jack since the condition is true. IT reached 11 so it was true. Why does it keep going to the next 'else if'?
5 Answers

J.D. Sandifer
18,813 PointsIn case this helps you understand better, here's my answer:
Look at the two parts to your code: 1) the array and for loop, and 2) the stuff in the for loop's curly brackets
Array and For Loop
let cards = 1...13
for card in cards {
// this loop will run 13 times, once for each of the 13 numbers in the cards array
// whatever code is in here happens 13 times
}
The Stuff in the For Loop
// every time this code runs it will print out either
// Jack, Queen, King, or a number (the value of card)
if card == 11 {
println("Jack")
} else if card == 12 {
println("Queen")
} else if card == 13 {
println("King")
} else {
println(card)
}
// because it's in the for loop above, it will run 13 times
Hope that helps!
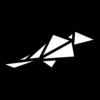
B B
2,934 PointsIt looks like you created an array of cards, so its going through each card, one will be 11, one will be 12, one will be 13, etc. so each of these lines should print on their individual card.
11 prints jack on its turn 12 prints queen on its turn
etc.

tytyty
4,974 PointsYes, thanks. That is the easy part for me to grasp. I'm confused why it goes through each card. It is supposed to stop when the condition is true and there is a card 11 which means the condition has been met so it should stop. Why does it continue through the array?
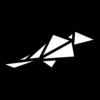
B B
2,934 PointsBecause you are doing a for loop so its doing that loop on each one of the cards individually
for card in cards tells the system that you want to run the following code on each one of the cards in the array.
card 11 runs through until true or fails
card 12 runs through until true or fails... etc.

tytyty
4,974 PointsBut again Brett you said "card 11 runs through until true or fails". UNTIL is the key word I'm talking about. It was 11/ true therefore it should have stopped according to what you said. Or until it failed (i.e. there is no number 11). But it keeps going to 12,13. I understand the function of the loop and array but am confused about the logic of the else if. In my understanding it is supposed to stop at 11 since it's true (even though its a loop).
I think what's happening is I'm misinterpreting an else if statement as "or else" in English.

J.D. Sandifer
18,813 PointsTymac, did you see my answer above? The loop is independent of the if/else statements. The loop happens 13 times regardless of the result of the if/else's.
The "stopping" that might be confusing you is that when the first if statement is true, then it "stops" and doesn't go to the else if or else statements. The program still goes through the loop again, though, because none of the if statements cause a change in the variable card - which is controlling how the loop works.
You're semantic understanding of else if seems right: The program goes through the if/else statements and does the first one if it's true, or else it goes on to the second one and does it if it's true, and so on or else it does the final else statement if nothing previous was true.

tytyty
4,974 PointsYup saw it. Ok I think the problem was that I didn't realize the program still goes through the loop. All this time I thought if statements also control loops. For example if the condition is true the loop will stop.
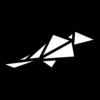
B B
2,934 PointsYou are right, a loop does stop once the condition is met, you have a loop within a loop though, so it just goes to the next card.
[for card in cards] <---- your first loop, this loop runs 13 times, once for each card
[if card] <-- your second loop within your for loop.
its basically like setting 13 cards down on the table, saying that you want to do a check on each one of them individually and then proceeding to pick up a card and ask yourself if its 11 then you know its a jack. Okay you are done identifying that one, on to the next card... so on.

tytyty
4,974 PointsYou mean like a dream within a dream? lol You know I had to use that one ;)
Ohhhh I thought it was like one loop in total so I didn't understand why it would keep going. But it's actually one loop for each number.
Totally messed me up. I just couldn't get it.
Thanks Brett && J.D.